Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial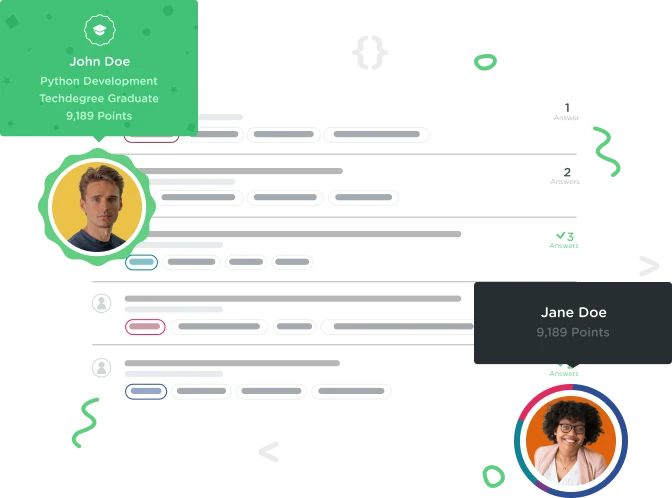
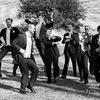
Jonathan Whittle
28,309 PointsHelp with the DOM
I need to set the text content of the a (<a>?) tag to the inputValue var. Can someone please help me understand how to go about doing this?
let inputValue = document.querySelector('a').textContent;
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<div id="content">
<label>Link Name:</label>
<input type="text" id="linkName">
<a id="link" href="https://teamtreehouse.com"></a>
</div>
<script src="app.js"></script>
</body>
</html>
1 Answer
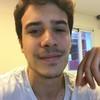
Ignacio Martin Elias
10,127 PointsThe challenge its telling you to set the text content of the a tag with the value you have already stored in the variable inputValue. So you need first to select the only a tag element there is. And then you need to change its text content with the value stored previously in the inputValue variable. It should be something like this.
let inputValue = document.querySelector("input").value;
const a = document.querySelector("a");
a.textContent = inputValue;
Hope it helps!
abcdefte
25,162 Pointsabcdefte
25,162 PointsHey so from the code you posted I see an input and an anchor tag in the html :
If I'm understanding your question correctly you want to use the input value as the anchor tag's text? if so it would look like this: