Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial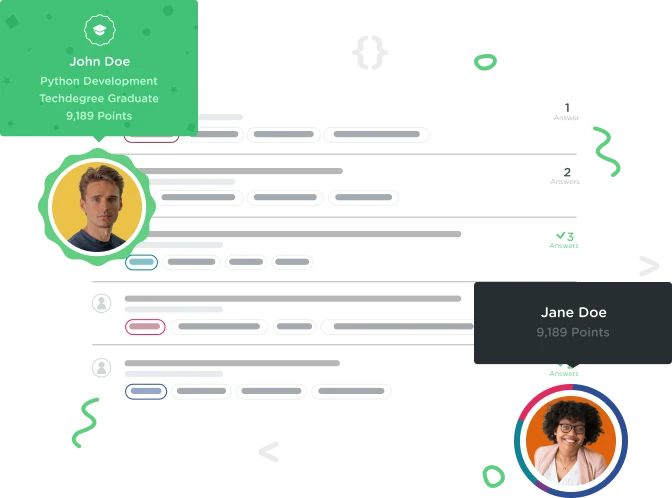

Chris Kopcow
2,852 PointsHelp with the Interfaces Code Challenge
I feel like the answer to this question will be a super-obvious detail I've missed, but after trying to figure it out for a while, I felt like I should ask.
On the second task of the Interfaces code challenge, it asks me to implement an interface and make it so the Perform method increments the value passed in by 1. I wrote this all out, and it compiles, but Treehouse keeps asking me "Does Increment have a constructor that takes no parameters?" whether I have an Increment constructor with no parameters or not.
Comparing what I've written to other similar code that implements interfaces, it doesn't seem like I'm doing anything wrong, unless I'm misinterpreting what Treehouse is asking me. Does anyone know what's going on?
namespace Treehouse.CodeChallenges
{
interface IUnaryOperation
{
double Perform(double value);
}
}
namespace Treehouse.CodeChallenges
{
public abstract class Increment : IUnaryOperation
{
public Increment()
{
}
public double Perform(double value)
{
return value++;
}
}
}
1 Answer
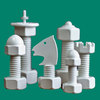
Steven Parker
231,269 PointsThe message is perhaps misleadng. But the issue is that a class that implements an interface and can be instantiated would not be an abstract class.
Also, you've used the post-increment operator, which will return the value prior to incrementing. All you need here is ordinary addition, or you could use the pre-increment operator (two plus signs in front).
Chris Kopcow
2,852 PointsChris Kopcow
2,852 PointsOkay, so I took out
abstract
from the class, and it worked! Thanks!But I'm not sure I understand why. In the code for the TreehouseDefense defense game you make for the class, there's an abstract Invader class that implements the IInvader interface and also has its own constructor (though it takes a parameter). Why is that class allowed to be abstract?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsCan you provide a link or a course page or workspace snapshot so I can take a look at the code in question?
Chris Kopcow
2,852 PointsChris Kopcow
2,852 PointsSure! https://w.trhou.se/3iq7g94z56
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThe abstract "Invader" class does implement the "IInvader" interface, but it is never instantiated in the game. It is only used as the base class for several different variations that get instantiated.
It would not make sense to create an abstract class unless one or more classes that inherit from it are also created.
Chris Kopcow
2,852 PointsChris Kopcow
2,852 PointsOhhhh, right, right. I see. I think I got mixed up and thought that a class had to be abstract to implement an interface for some reason. Thanks so much!