Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial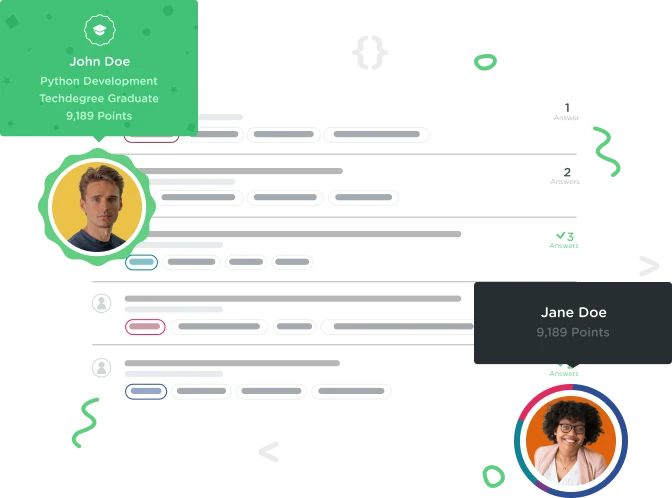
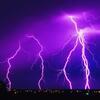
Janelle Mackenzie
7,510 PointsHelp with the newDueDate method
I am having quite a hard time understanding the logic behind the solution code used in the course. And I would like to know if my code would work as well (to me it seems more simple and clean) and I can't find to get my answer by logging either one to the console as it just repeats the function and doesn't run the function... Can someone please tell me if/where I went wrong and explain the solution code? Also how come when I log my 'dueDate' function to the console it only gives me the javascript code I wrote rather than actually running the function?
// SOLUTION CODE
const newDueDate = new Date();
newDueDate.setDate(newDueDate.getDate() + 14);
book.dueDate = newDueDate;
//MY CODE
const dueDate = function() {
let currentDate = new Date();
currentDate.setDate(currentDate + 14);
}
3 Answers

Mike Straw
7,100 PointsHi Janelle Mackenzie ,
I'm not sure if Simon Coates' answer worked for you or not, since there wasn't anything selected as the "Best answer", so I thought I'd take a stab at it, too :)
Can someone please tell me if/where I went wrong and explain the solution code?
The key is what's being set. The solution code does this:
newDueDate.setDate(newDueDate.getDate() + 14);
You'll see that is first calls the getDate()
method. This gets the current date, then newDueDate
's date value is set to 14 days after that.
In your function, the string '14' is appended to the object literal currentDate
.
currentDate.setDate(currentDate + 14);
Objects don't have values in and of themselves, so you have to use the currentDate object's properties to get its date value. Something like this would work:
currentDate.setDate(currentDate.getDate() + 14);
Also how come when I log my 'dueDate' function to the console it only gives me the javascript code I wrote rather than actually running the function?
I suspect your console.log
call looks something like this:
console.log(dueDate);
When it should be like this:
console.log(dueDate());
The ()
is the key: without the parentheses, console.log
returns the function itself, so you see the code of the function. Adding the parentheses tells it to call the function, then display the returned value.
I hope this helps!

Simon Coates
8,481 PointsI copied your code and it ran when I used dueDate(). It just didn't do anything as the variable only exists for the method call. It isn't passed in or returned. Additionally, I tried your means of setting the date and got a result of Invalid Date. The results of examining it in the console are below:
const dueDate = function() {
let currentDate = new Date();
currentDate.setDate(currentDate + 14);
}
//undefined
dueDate();
//undefined
let currentDate = new Date();
//undefined
currentDate.setDate(currentDate + 14);
//NaN
currentDate;
//Invalid Date
The comments are the output via the console.
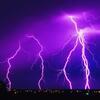
Janelle Mackenzie
7,510 PointsSooooooo.. that means what? lol

Simon Coates
8,481 PointsIf you're using the setDate method incorrectly, them you should refine your understanding of what it accepts and what you're attempting to pass in. I looked at https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date/setDate and it seems like the method accepts a displacement in days. In terms of your function, you could do something like:
const updateDueDate = function(aDate) {
aDate.setDate(aDate.getDate() + 14);
}
//undefined
var today = new Date();
//undefined
updateDueDate(today);
//undefined
today;
//Thu Nov 05 2020 23:51:26 GMT+1000 (Australian Eastern Standard Time)
or
const updateDueDate = function(aDate, displacement) {
aDate.setDate(aDate.getDate() + displacement);
}
//undefined
var today = new Date();
//undefined
updateDueDate(today, 14);
//undefined
today;
//Thu Nov 05 2020 23:52:58 GMT+1000 (Australian Eastern Standard Time)
Update: I think I was wrong about the cause of the problem. While an excessively large number would crash setDate, I think the problem is that currentDate + 14 in your code may convert to a string. I assumed it would convert to an integer.

Simon Coates
8,481 PointsI revised my comment to eliminate a faulty understanding on my part (sorry). The other way to use a function to create a dueDate might be something like
const getDueDate = function(displacement) {
let aDate = new Date();
aDate.setDate(aDate.getDate() + displacement);
return aDate;
}
var someDate = getDueDate(14);
someDate;
//Fri Nov 06 2020 00:35:11 GMT+1000 (Australian Eastern Standard Time)
Because setDate alters the value of the date object (ie. it's destructive rather than returning a copy), you need to either pass in a date for the function to alter or receive a date back from the function.
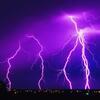
Janelle Mackenzie
7,510 PointsSimon Coates I appreciate your effort to explain! But I'm still having trouble relating your explanation to the code provided by the solution in my original post.. I still don't understand why my code doesn't work and I also don't understand why the one above mine does...

Simon Coates
8,481 PointsThe first batch of code creates a data, updates the date and then uses the date (assigning it to an attribute on a variable). It's stored. The function you wrote doesn't accept a date and doesn't return a date. It does HAVE a date, but after it alters it (in a flawed way), it doesn't do anything with it. The function executes and does nothing. The versions of the function I wrote either pass in a date to modify (the parameter aDate in the function definition) or pass out a modified date (the return statement). The date exists outside the function.
Because you define the variable currentDate inside a function (and used the let keyword), it's a local variable. Unless you takes further steps, that variable is only accessible inside the function. So with:
var book = {};
const newDueDate = new Date();
newDueDate.setDate(newDueDate.getDate() + 14);
book.dueDate = newDueDate;
//Fri Nov 06 2020 01:34:07 GMT+1000 (Australian Eastern Standard Time)
book.dueDate;
//Fri Nov 06 2020 01:34:07 GMT+1000 (Australian Eastern Standard Time)
newDueDate;
//Fri Nov 06 2020 01:34:07 GMT+1000 (Australian Eastern Standard Time)
I can access the variable. But with:
const dueDate = function() {
let currentDate = new Date();
currentDate.setDate(currentDate + 14);
}
dueDate();
currentDate;
//VM469:1 Uncaught ReferenceError: currentDate is not defined at <anonymous>:1:1
trying to access the variable outside the function produces an error. (there's a bit of an explanation of 'scope' in the treehouse javascript functions course or a very simplistic discussion of local variables at https://www.w3schools.com/js/js_scope.asp .)
Joe Elliot
5,330 PointsJoe Elliot
5,330 PointsThanks Mike, I always accidentally log the function by not putting the parenthesis in order to call it!