Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial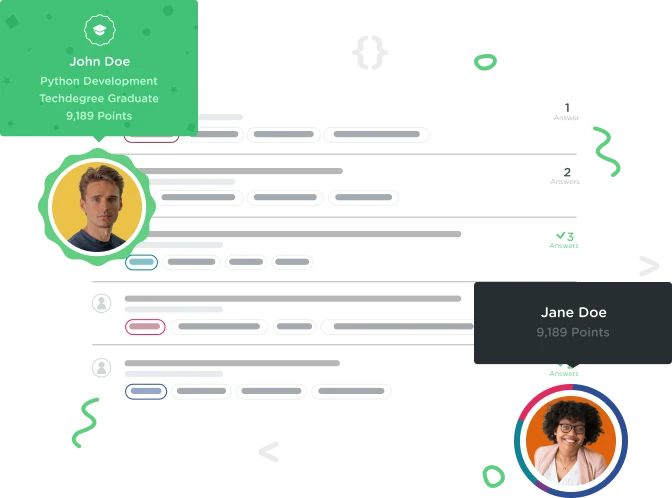
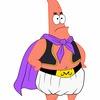
<noob />
17,062 PointsHelp with this challange
What is wrong with my code?
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static int[,] BuildMultiplicationTable(int maxFactor)
{
int[,] sheet = new int[maxFactor][];
for(int rowIndex = 0; rowIndex < sheet.Length; rowIndex++)
{
for(int colIndex = 0; colIndex < sheet[rowIndex].Length; colIndex++)
{
sheet[rowIndex,colIndex] = rowIndex * colIndex;
}
}
}
}
}
1 Answer
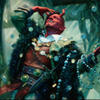
Daniel Medina
13,863 PointsHello bot,
There are a few changes you can make to your code.
Instead of new int[maxFactor][]
, try this:
int[,] sheet = new int[maxFactor, maxFactor]; // format: x, y
Your first idea of using .Length makes sense given that this is
an array. However, for multidimensional arrays, try using the .GetLength
method. So sheet.GetLength(0)
for your first dimension,
sheet.GetLength(1)
for your second dimension, etc.
Lastly, don't forget to return sheet outside of the for loops.
Other than these small tweaks, it looks great. Keep it up!
Some quick articles I found:
StackOverflow: https://stackoverflow.com/questions/9301109/how-do-you-loop-through-a-multidimensional-array
MSDN: https://docs.microsoft.com/en-us/dotnet/csharp/programming-guide/arrays/multidimensional-arrays