Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial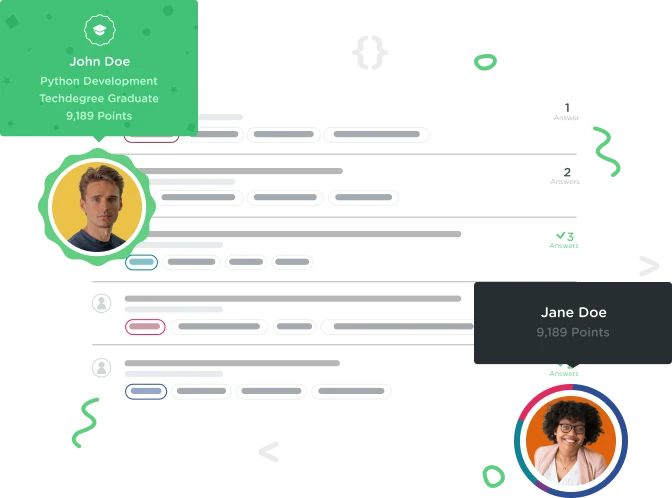

Jessica Sharp
4,018 Pointshelp with this challenge
i get an attribute error
def disemvowel(word):
word.remove(a,e,i,o,u,A,E,I,O,U)
return word
1 Answer
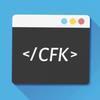
Aayush Mitra
24,904 PointsHi Jessica!
You have a few errors.
First of all, you are treating the "word" argument like a list. Also you need to wrap the letters with quotation marks because it is a string.
Okay, so lets start from a clean plate. First of all, you will need to create a variable with all of the vowels, upper and lowercase, which you can use later.
Next, you need to change the "word" or string to a list. This is so that you can use this later too.
Then, you need to make a for loop that will loop through all of the letters in the word. Your code should look like this by now:
def disemvowel(word):
upper_and_lowercase_vowels = "aeiouAEIOU"
the_list = list(word)
for letter in word:
So in the loop, we need to ask if the letter is in or "is" one of the vowels, then remove that letter from the "the_list" variable. The if statement should look like this:
if letter in upper_and_lowercase_vowels:
the_list.remove(letter)
Finally, we need to make sure that all of the letters are still close beside eachother, otherwise it would not be a word! We have been making all our changes to the "the_list" variable, so now that we are done, we need to return the word variable, so we need to turn the word variable into the "the_list" variable. Like this:
word = ''.join(the_list)
Here is the final code:
def disemvowel(word):
upper_and_lowercase_vowels = "aeiouAEIOU"
the_list = list(word)
for letter in word:
if letter in upper_and_lowercase_vowels:
the_list.remove(letter)
word = ''.join(the_list)
return word
One more thing, next time when you are having a problem with your code, I think you should probably re-watch the video two or three times, before jumping to the community. If that does not work, then I would recommend going to the community and instead of asking, looking for a question that matches yours and try and understand why the answer is what it is. And also, the chances of other people running into the same problem as you is very high. What this will do is allow you to be more independent. If you absolutely must, then you should post on the forums.
Anyway, hope that works.
If it does not please tell me.
Thanks! :)
Kip Yin
4,847 PointsKip Yin
4,847 PointsWell, first off,
word
is a string, so it doesn't have.remove()
method. Try to convert it to a list first.Secondly, if you don't add quote marks to
a, e, i, o, u
, etc., Python will treat them as variables, not strings, hence theAttributeError
.Also, the
.remove()
method takes exactly one argument, which is the value to be removed from the list. You have 10 arguments in.remove()
.Lastly, I think no matter how you do this challenge, you need to use some kind of loop (unless you use more advanced functions). E.g., loop through all the letters in the input string, then remove that letter if it's a vowel, and finally return the filtered string.