Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial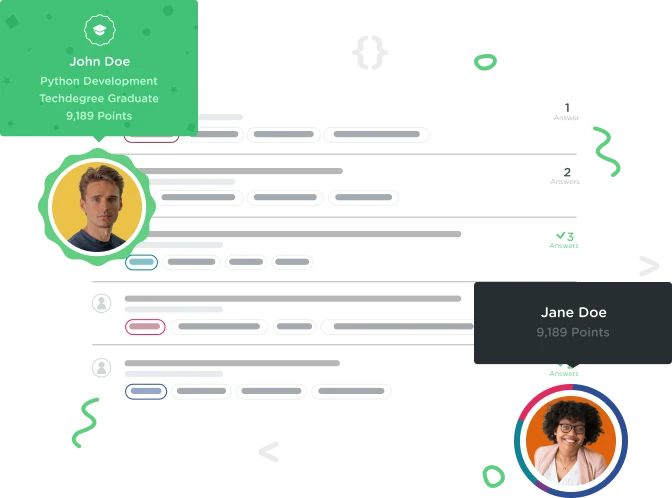
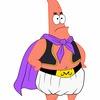
<noob />
17,062 Pointshelp with this challnage
i get this error: Whoops looks like you didn't censor the words (2a). mCensoredWords contains 'dork', but it made it past somehow.
the code:
main:
package com.teamtreehouse;
public class Main {
public static void main(String[] args) {
// write your code here
Prompter prompter = new Prompter();
System.out.printf("Please give me a story, with placeholders in between double underscores%n");
System.out.printf("(e.g. '__name__', '__adjective__', '__noun__'): %n");
String story = prompter.promptForStory();
Template tmpl = new Template(story);
prompter.run(tmpl);
}
}
prompter:
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
//creating a new Set to store the censored words from the censored_words.text file.
mCensoredWords = new HashSet<String>();
//here im creating a access point to the censored_words file.
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
//we "read" all the content from the file with the Files.readAllLines object and store the content in the "words" List.
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
//now i add all the censored words from the "words" list(the list contain the censored words from the txt file) to the Set(mCensoredWords)
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> wordsResult = null;
try {
wordsResult = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
String result = tmpl.render(wordsResult);
System.out.printf("Your TreeStory:%n%n%s", result);
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) throws IOException {
System.out.printf("Please enter your word for %s %n", phrase);
String entryWord = mReader.readLine();
while (mCensoredWords.contains(entryWord.toLowerCase())) {
System.out.printf("%s is not a valid word!, please Try again.. %n", entryWord);
entryWord = mReader.readLine();
}
return entryWord.trim();
}
public String promptForStory() {
String story = null;
try {
story = mReader.readLine();
} catch (IOException e) {
e.printStackTrace();
}
return story;
}
}
Tonnie Fanadez any help with this? can u try to run the code? when i run this it did blocked dork
package com.teamtreehouse;
public class Main {
public static void main(String[] args) {
// write your code here
Prompter prompter = new Prompter();
System.out.printf("Please give me a story, with placeholders in between double underscores%n");
System.out.printf("(e.g. '__name__', '__adjective__', '__noun__'): %n");
String story = prompter.promptForStory();
Template tmpl = new Template(story);
prompter.run(tmpl);
}
}
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
//creating a new Set to store the censored words from the censored_words.text file.
mCensoredWords = new HashSet<String>();
//here im creating a access point to the censored_words file.
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
//we "read" all the content from the file with the Files.readAllLines object and store the content in the "words" List.
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
//now i add all the censored words from the "words" list(the list contain the censored words from the txt file) to the Set(mCensoredWords)
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> wordsResult = null;
try {
wordsResult = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
String result = tmpl.render(wordsResult);
System.out.printf("Your TreeStory:%n%n%s", result);
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) throws IOException {
System.out.printf("Please enter your word for %s %n", phrase);
String entryWord = mReader.readLine();
while (mCensoredWords.contains(entryWord.toLowerCase())) {
System.out.printf("%s is not a valid word!, please Try again.. %n", entryWord);
entryWord = mReader.readLine();
}
return entryWord.trim();
}
public String promptForStory() {
String story = null;
try {
story = mReader.readLine();
} catch (IOException e) {
e.printStackTrace();
}
return story;
}
}
# This is essentially what I am testing
1. The user is prompted for a new string template (the one with the double underscores in it).
a. The prompter class has a new method that prompts for the story template, and that method is called.
2. The user is then prompted for each word that has been double underscored.
a. The answer is checked to see if it is contained in the censored words.
User is continually prompted until they enter a valid word
3. The user is presented with the completed story
9 Answers

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsThe app that you will build in JavaFx has an interesting logic that I liked. However, halfway through JavaFx it turned hard for me because of CSS and FXML which I don't have experience in; so I just brushed through it and finished the course.
Just try the Treehouse JavaFx course first and if content is not enough you can always look elsewhere. I haven't heard about Boston but surely you will need a lot of Java before you can start Android. You are on the right course noob developer; myself I have done Android and it is the best course ever. From Monday I will be studying a TechDegree in UX.
In relation to the challenge while-loop works just fine on my IDE it doesn't allow words like "dork" so I think it is a bug like you said. Anyway, the way I got around it was to use a do-while loop instead. I have pasted the code for you above.
Please see this link on how guys approached the same challenge .
https://teamtreehouse.com/community/i-completed-this-challenge-any-feedback.
Cheers

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsGreat work man!!
Just try do-while loop in promptForWord () method and tell me how it goes. The other code looks fine to me.
Cheers

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsMy Code for your reference.
noob developer remember to use the phrase parameter provided on the promptForWord () method.
public String promptForWord (String phrase) throws IOException {
do {
word = mReader.readLine();
if (mCensoredWords.contains(word.toLowerCase())) {
System.out.printf("%s is not allowed, please try again", word);
System.out.printf("Enter %s", phrase);
}
} while (mCensoredWords.contains(word));
return word;
}
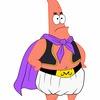
<noob />
17,062 Pointsthanks! i will try it i’m not at the computer right now. u didn’t see any errors?, it was kinda hard to figure it out so i saw other people’s code and began to understand. why we intitalize a template?
another question i have is this, i saw you have knowdelge in javafx , do u go course by course or u jump?
btw since ur very active and in too, would u like to talk in a discord channel or something that will be faster

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsMorning noob developer
Just try when you get to your computer. The code was a bit hard and it took me time to figure it out. The only thing I noted about your code is that you used while loop instead of do-while and that's why you are getting the error.
Sure we can talk on Discord (look me up @Fanadez) . I am a beginner student in both Java and JavaFx and have just finished JavaFx course on Tree house and I can say it is tougher than the normal Java. In Fact I would rather do Android instead. You have done HTML, CSS, Python and Java Script--Congrats! I think you will just cruise through it.
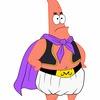
<noob />
17,062 PointsLol i started from js then tried python , then c#, i find the c# track pretty hard if u don’t have an oop knowledge and that’s why i moved to the java track which turn to be very good, i think when i’m done with intermediate track i’ll continue to the java web dev and then to android, it is better no? btw the new boston have a toturials on javafx on youtube, i didn’t try them yet because i don’t got there but u should check it out.
about th challnage in the ide it’s wokkng as intended, it blocks the word that’s why i think there is a bug
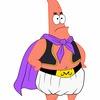
<noob />
17,062 Points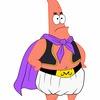
<noob />
17,062 Pointsbtw, javafx course what fun? it looks awesome!!
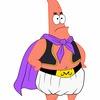
<noob />
17,062 Pointsi tried :
public String promptForWord(String phrase) throws IOException {
String entryWord;
do {
System.out.printf("Please enter your word for %s %n", phrase);
entryWord = mReader.readLine();
if(mCensoredWords.contains(entryWord.toLowerCase())){
System.out.printf("%s is not a valid word!, please Try again.. : %n", entryWord);
System.out.printf("Enter %s", phrase);
}
}while(mCensoredWords.contains(entryWord));
return entryWord;
}
same error.. its wworking in the ide though. can u paste the complete code?
EDIT*****: Turns out we dont need the if statment tonnie, somehow it passed without it.

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 Pointspackage com.teamtreehouse;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
Prompter prompter = new Prompter();
Template tmpl = null;
try {
tmpl = new Template(prompter.promptforNewStoryLine());
} catch (IOException e) {
e.printStackTrace();
}
prompter.run(tmpl);
}
}

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 Pointspackage com.teamtreehouse;
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.util.ArrayList; import java.util.HashSet; import java.util.List; import java.util.Set;
public class Prompter {
String word;
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
String result = tmpl.render(results);
System.out.printf("Your TreeStory:%n%n%s", result);
}
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
public String promptForWord(String phrase) throws IOException {
String word;
System.out.printf("Please enter the %s: ", phrase);
boolean goodResponse = false;
do {
word = mReader.readLine();
if (!mCensoredWords.contains(word)) {
goodResponse = true;
}
} while (!goodResponse);
return word;
}
public String promptforNewStoryLine() throws IOException {
System.out.println("Enter a Storyline");
String storyLine = mReader.readLine();
return storyLine;
}
}