Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial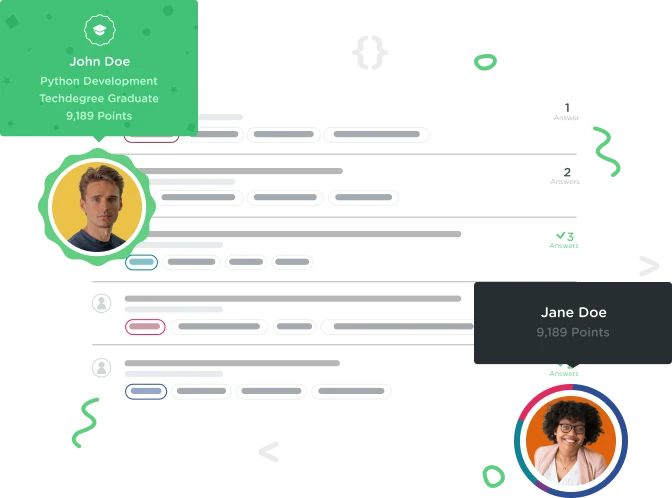
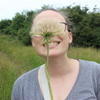
Ioana Rusu
9,599 PointsHelp with this code challenge :)
I really need some help with this code challenge as I don't think I understood exactly what and how.
This is the question :
Use the map method on the daysOfWeek array, creating a new array of abbreviated week days. Each abbreviated string should be the first three letters of the long version in daysOfWeek. Store the new array in the variable abbreviatedDays.
const daysOfWeek = ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"];
let abbreviatedDays;
// abbreviatedDays should be: ["Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat"]
// Write your code below
6 Answers
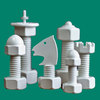
Steven Parker
230,274 PointsYou know from the instructions that they want you to use "map", and Marcin's given you a good example of the basic usage. You know how "map" works, right? For a brief recap, the argument you give to "map" is a callback function. And the function should take an argument and return whatever you want done with it.
So in addition to the basic structure for calling "map", you will write a function that takes a string argument and returns the first three letters from that string. There are many ways to do that, but "slice" might be handy.
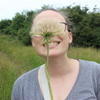
Ioana Rusu
9,599 PointsHi,
I have tried and tried and I cannot figure out that should I write exactly so I can have the result. I must sound weird and stupid but this is totally new to me, never heard of slice() before. Can I have a little more help? Spent hours trying to figure out what is the code .....even if I had your help.
Thanks again
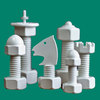
Steven Parker
230,274 PointsIf you'd like to practice using essential documentation, here's the MDN page for slice.
Otherwise, just disregard that suggestion entirely and use any method you like to get the first 3 letters from each string.
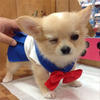
Kristiana Georgieva
14,595 PointsHey, guys!
After doing a bit of Googling myself, I ended up using the 'substring' method and I was able to pass the challenge with it as well. You can read more about it here.
I used it the following way:
const daysOfWeek = ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"];
let abbreviatedDays;
abbreviatedDays = daysOfWeek.map(day => day.substring(0, 3));
console.log(abbreviatedDays);
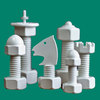
Steven Parker
230,274 PointsGood job! FYI: "substring" is almost exactly the same thing as "slice".

Aidan Lowson
10,379 PointsGreat solution! Steven Parker in this case day.slice(0, 3)
would return "Sunday", "Monday", "Tuesday".
There's some useful documentation in regards to this on the W3S website https://www.w3schools.com/jsref/jsref_slice_array.asp
Also on Mozilla's MDN: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/slice
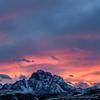
Jason Dsouza
1,378 PointsThis really helped. Never knew what slice() meant. Guess i just learned something new. Thanks mate!
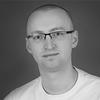
Mateusz Machnik
10,594 PointsabbreviatedDays = daysOfWeek.map(day => day.slice(0,3))
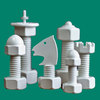
Steven Parker
230,274 Points Explicit answers without any explanation are strongly discouraged by Treehouse and may be redacted by moderators.
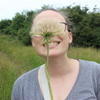
Ioana Rusu
9,599 PointsHi Steven,
I have looked into MDN, always do, I was just confused about how the code supposed to look like and I have decided to check another method to get the first 3 letters from each string and I did it in the end.
Thank you so much for your help :)
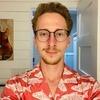
David Shulkin
Front End Web Development Techdegree Student 10,254 PointsHi all! Just wanted to share my solution to the code challenge. What I did here is assign the function to the variable 'days' that way abbreviatedDays can be reused somewhere else if necessary.
const daysOfWeek = ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"]
const days = day => day.substring(0, 3)
const abbreviatedDays = daysOfWeek.map(days)
console.log(abbreviatedDays)
Marcin Czachor
9,641 PointsMarcin Czachor
9,641 PointsHey
There is Array.prototype.map() method build in JS Array object. It means that every array inherits that method and it can be used on every array. In this example you should use this method on daysOfWeek and store result in abbreviatedDays variable.
You can find more info about Array.prototype.map() method on MDN. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map