Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial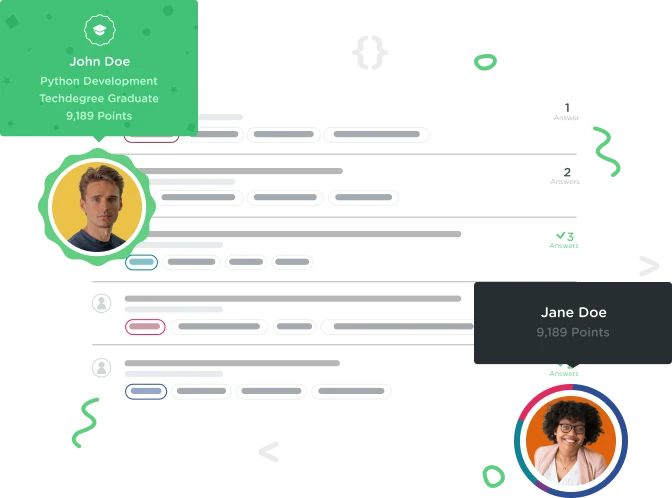

chadtrujillo
3,013 PointsHelp with tuples! Final section for Tuples is driving me insane!
I have tried so many different things and cannot seem to get this to come out right. I have tried adding tuples to the return but to no avail.
func greeting(person: String) -> (langugage: String, greeting: String){ let language = "English" let greeting = "Hello (person)"
return greeting("English", "Chuck Norris")
}
func greeting(person: String) -> (langugage: String, greeting: String){
let language = "English"
let greeting = "Hello \(person)"
return greeting
}
4 Answers
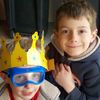
Chris Stromberg
Courses Plus Student 13,389 Points// This will only create a infinite loop when you pass the function back
// into the same function you are calling.
func greeting(person: String) -> (language: String, greeting: String) {
let xLanguage = "English"
let xGreeting = "Hello \(person)"
return greeting(xLanguage)
}
// Here your returning the greeting function within your code when you should only be returning a tuple.
func greeting(person: String) -> (language: String, greeting: String) {
let xLanguage = "English"
let xGreeting = "Hello \(person)"
return greeting(xLanguage, xGreeting)
}
// Here is what you want to do, just return a tuple with a string named language,
// and a string named greeting.
// Your function signature states you have a function called "greeting"
// it takes one parameter called "person" of type String
// it return two things (a tuple): a String called "language" and a String called "greeting".
func greeting(person: String) -> (language: String, greeting: String) {
let language = "English"
let greeting = "Hello \(person)"
return (language, greeting)
}
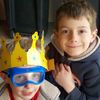
Chris Stromberg
Courses Plus Student 13,389 PointsYour function signature states that it will return a tuple.
However in your code you are only returning a greeting constant.
return should be: return ( language , greeting )

chadtrujillo
3,013 PointsI was able to get it to pass the first part by using
func greeting(person: String) -> (language: String, greeting: String) {
let xLanguage = "English"
let xGreeting = "Hello \(person)"
return greeting(xLanguage)
}
however if I try to do this it fails.
func greeting(person: String) -> (language: String, greeting: String) {
let xLanguage = "English"
let xGreeting = "Hello \(person)"
return greeting(xLanguage, xGreeting)
}
it really doesn't effect the course but I am curious why?

chadtrujillo
3,013 PointsI have to go back and re-watch this section i think. I am struggling with these tuples. I understand where I went wrong with what I was doing now seeing the correct way of doing it. Now I can't seem to create a variable holding the correct value.
Instructions are: Create a variable named result and assign it the tuple returned from function greeting. (Note: pass the string "Tom" to the greeting function.)
and here is what I thought was what I should do? In xCode this works right? On here I am getting an error that says "Bummer! Your result
variable has the wrong value in it. Check the task instructions again."
func greeting(person: String) -> (language: String, greeting: String) {
let language = "English"
let greeting = "Hello \(person)"
return (language, greeting)
}
var result = greeting("Tom")

chadtrujillo
3,013 PointsNever mind, not sure what the issue was but when I changed the return from the greeting function to have "greeting, language" instead of "language, greeting" it worked.
Julianne Lefelhocz
2,091 PointsJulianne Lefelhocz
2,091 PointsYou need to return greeting and language (make sure it is in the same order as after the -> also make sure language is spelled right :))
return (language, greeting)