Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial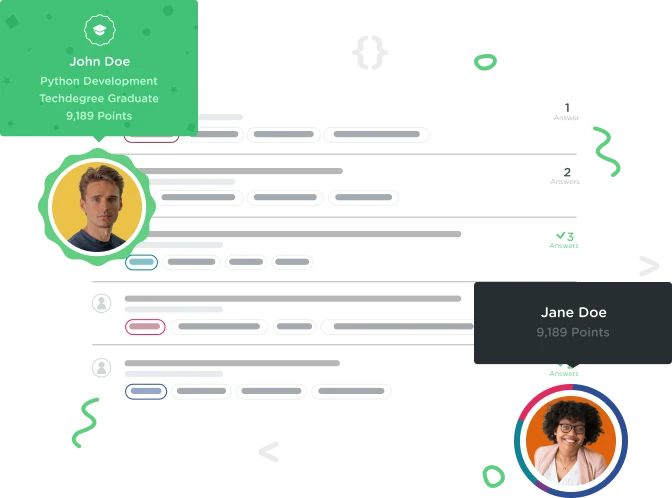

Kamen Mackay
2,179 PointsHelp with understanding tuples/variable assignments?
So my solution worked but, as I was working on this problem,I don't understand why 'foo' is not being unpacked and multiplied. Here is my function:
def multiply(*args):
product = 1
for arg in args:
product = arg * product
return product
First example:
foo = ( 1,2,3,4 )
multiply(foo)
multiply returns '(1,2,3,4)
Second example:
But if I feed the numbers directly into the function rather than using 'foo':
multiply(1,2,3,4)
multiply returns '24'
Can someone explain what's happening and what I'm doing wrong?
1 Answer
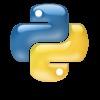
Viraj Deshaval
4,874 PointsYour code is correct. The only thing where you are doing mistake is calling a function with tuple. You call your function as multiply(5, 6, 8). Try this it will work.

Kamen Mackay
2,179 PointsI understand that but my question was how would I pass it as a variable and have it return the same result? I know that I passed this section but I'd like to understand some of the finer details so I can master Python.
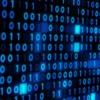
Alexander Davison
65,469 PointsTry:
def multiply(args):
product = 1
for arg in args:
product = arg * product
return product
(Note that there's no asterisk before the args
parameter)
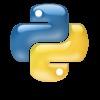
Viraj Deshaval
4,874 PointsGood question. Let me explain you what happened to the code you developed and why it didn't worked.
So when you execute your code i.e.
def multiply(*args): # args: <type 'tuple'>: ((1, 2, 3, 4), )
product = 1 # product: <type 'tuple'>: (1, 2, 3, 4)
for arg in args: # arg: <type 'tuple'>: (1, 2, 3, 4)
product = arg * product
return product
foo = (1,2,3,4)
print(multiply(foo))
what this does is you have a tuple named foo and whole tuple(((1, 2, 3, 4), ) got passed. Why? because if you see the functionality of tuple i.e. it will unpacks the values to the variables available in left side, but in your case only single variable so whole tuple (1,2,3,4) is equal to foo. so now if we loop through this the program will return the product = (1,2,3,4) instead of 24.
To resolve this what you need to now think is how you can pass the values as multiply(1, 2, 3, 4) instead of multiply((1, 2, 3, 4), ). Let's solve now your query. In order to pass the values from tuple variable follow below steps: Step - 1 : First of all you need to pack the tuple variable i.e multiply(*foo) Step - 2: Then you pass the packed tuple to function and then function will loop through each values inside tuple. See below what I mean.
def multiply(*args): #args: <type 'tuple'>: (1, 2, 3, 4)
product = 1 #product: 24
for arg in args:
product = arg * product
return product
foo = (1,2,3,4)
print(multiply(*foo))
In this way you can pack and unpacks the tuple.
HappyCoding
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsPlease format your code properly so that people can read it. Refer to the Markdown Cheatsheet below the answer box
It says: