Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial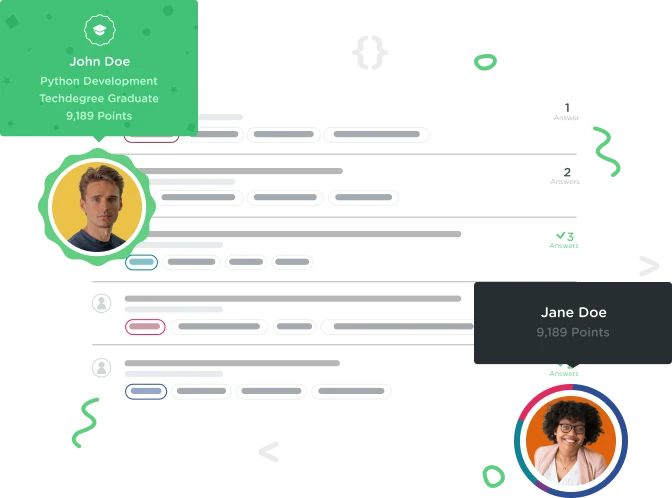

Scott James
4,298 PointsHelp with "Working with Functions" quiz.
Hi
I am working through the quiz, but I am stuck on a particular question, and wondered if someone could walk me through the question? Here is the code:
$numbers = array(1,2,3,4);
$total = count($numbers);
$sum = 0;
$output = "";
$i = 0;
foreach($numbers as $number) {
$i = $i + 1;
if ($i < $total) {
$sum = $sum + $number;
}
}
echo $sum;
I did copy the code in to my editor to get the answer, to then see if I could almost reverse engineer it, but I still can't quiet workout how to get to the value.
Any help on this is much appreciated :)
Thanks
9 Answers

Andy Bell
6,804 PointsThis question is asking what the code displays, so we need to take a look and see what is going to be echo-ed. You can see there's only one thing being echo-ed: the value of $sum at the very end of the code.
The foreach block is where we're going to get our final value for $sum, so let's determine what the foreach does. Here's the entire foreach:
foreach($numbers as $number) {
$i = $i + 1;
if ($i < $total) {
$sum = $sum + $number;
}
}
Breaking this down, each time we go through the foreach, $sum will be equal to the the previous $sum + the current number from the numbers array. However, we're also using a counter. If the counter ($i) is less than $total, we'll do the sum. If it is greater than **or equal to** $total, we won't.
$total counts the $numbers array. $total equals 4, because there are 4 items in the array. So we will actually go through the if only 3 times. So as we go through the foreach loop, here's what $sum and $i will equal:
- $i = 0 + 1 = 1; $sum = 0 + 1 = 1
- $i = 1 + 1 = 2; $sum = 1 + 2 = 3
- $i = 2 + 1 = 3; $sum = 3 + 3 = 6
- $i = 3 + 1 = 4; The if containing the sum code won't run this time because $i = 4 which is not less than $total (4). So $sum stays the same: 6.
So when we echo $sum at the end, it'll display 6!

Gordon Jiroux
8,728 PointsIt was my pleasure...
With respect to your next question,
It's because when you are concatenating the string in this method, you are pre-pending the number (adding it to the beginning of the string)
$output = $number . $output; (pre-pending)
is different than
$output = $output . $number; (adding it to the end)

Scott James
4,298 PointsHi Andy
So how comes when there is 4 items in the array, we will only go through the foreach loop 3 times? Is it because the first item in the array will be 0?
Thank you so much for the reply. That really cleared a lot up.

Gordon Jiroux
8,728 Pointsforeach will loop as many times as there are items in the array (barring anything that would "break" or exit execution of the loop)
however...
When $i is no longer less than $total, the condition is no longer able to be met to hit the code inside the "if". For instance, when 2 < 3, the condition ($var1 < $var2) is met. if 3 = 3, then $var1 is no longer less than $var2, and the condition isn't met.

Scott James
4,298 PointsHi Gordon
That makes sense. Why is it as soon as some one points it out, you think to yourself "of course that's what it means".
Can someone also help me on this quiz question?
<?php
$numbers = array(1,2,3,4);
$total = count($numbers);
$sum = 0;
$output = "";
$i = 0;
foreach($numbers as $number) {
$i = $i + 1;
if ($i < $total) {
$output = $number . $output;
}
}
echo $output;
?>
How does this echo out 321 in that order?
Thanks to everyone for the help :)

Scott James
4,298 PointsHi Gordon
I am having a lot of Aha moments today! lol Seriously thank you to every one that has helped out on this thread. Slowly but surely I feel like I am understanding PHP a bit better!

Gordon Jiroux
8,728 PointsNo problem. Scott...

Gordon Jiroux
8,728 Pointshere's a little shortcut when concatenating (to the end)
$output .= $number;
is the same as
$output = $output . $number;

Scott James
4,298 PointsNice tip Gordon. I will have to start using that one, to get in to the habit!
Thanks
Matthew Dixon
Courses Plus Student 3,783 PointsMatthew Dixon
Courses Plus Student 3,783 Pointswhy does the sum value increase?
Matthew Dixon
Courses Plus Student 3,783 PointsMatthew Dixon
Courses Plus Student 3,783 PointsNevermind it just clicked!
Every time the code executes the value of sum changes aka
for the first loop sum = 1 and the second loop sum = 3