Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial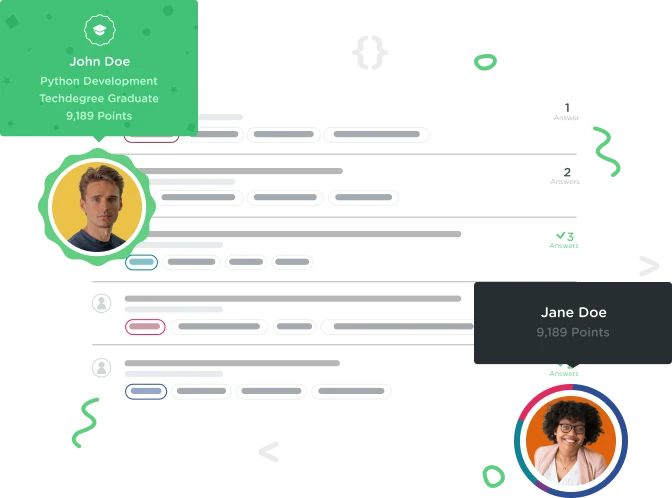

Sippi Software
6,648 Pointshelpme
plss help me
// Complete the challenge by writing JavaScript below
const colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
let listItems;
for ( let i = 0; i < colors.length; i++ ) {
listItems[i].style.color = colors[i];
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>JavaScript and the DOM</title>
</head>
<body>
<h1>My List</h1>
<ul>
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="app.js"></script>
</body>
</html>
2 Answers
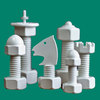
Steven Parker
230,274 PointsYou need to add an assigment to the declaration on line 3. You may want to review the Select Elements by Tag Name video lesson at pay special attention to the examples of getting an element using its tag name.

eotfofiw
Python Development Techdegree Student 823 PointsLine #2 contains a javascript array. This array is being iterated over in the for loop on line #5. The iteration of that array happens by accessing each item in the array by the index of value i
as you can see in colors[i]
That same notation is being used to assign a value to the listItems
collection with listItems[i].style.color
. The issue is that in the code above, listItems
has been declared, but not assigned any value. So the code is trying to assign to undefined
which isn't going to work.
You will need to use methods found on document to get a collection of elements in the HTML that you want to assign the color values to, and assign that to listItems
.
Once you have that, listItems
will contain a collection of objects that have a style property, and a you can set their color property correctly.