Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial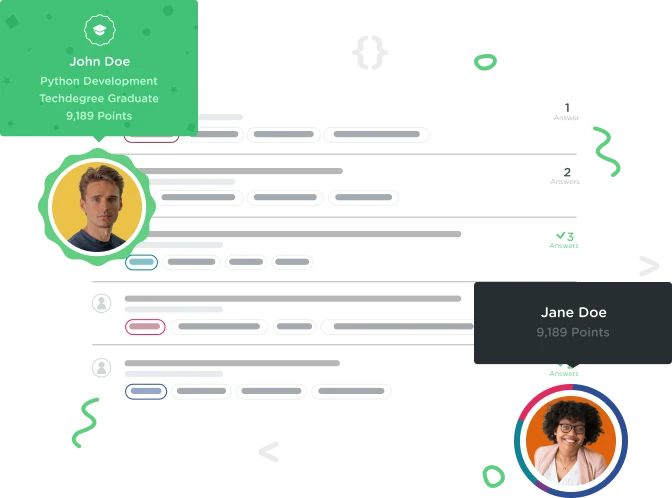

Adrian Thomas
1,572 PointsHere are my results for the first part of this challenge.
Ok, here is my results for this challenge:
// Questions & Answers
var questionsAnswers = [
[prompt("How many states are there in the United States?"), '50'],
[prompt("Out of the 50 states, which state is the largest of them all?"), 'Texas'],
[prompt("Which state is the smallest in the U.S.?"),'Rhode Island'],
[prompt("Which state is the coldest in the U.S.?"), 'Alaska'],
[prompt("Which state has the most population density in the U.S.?"), 'New Jersey']
];
var correct = 0;
var incorrect = 0;
function print(message) {
document.write(message);
}
// keeping score
function playerTotals (totals) {
for (var i = 0; i < totals.length; i += 1) {
if (totals[i][0] === totals[i][1]) {
correct += 1;
} else {
incorrect += 1;
}
}
}
// player results
function playerResults () {
document.write("<p>Ok, Here's how you did. You got " + correct + " answers right!</p>");
}
playerTotals(questionsAnswers);
playerResults();
What was really confusing me was the playerTotals
function that I've written, but now it makes sense. The function is comparing the player input with the answer. I thought it was comparing the question against the answer, but then I remembered that prompt
returns the input from the user.
Dave's results were completely different and I guess... simpler?
What do you think? How could I improve?
David Bath
25,940 PointsDavid Bath
25,940 PointsI think it's a bit confusing that you have the prompt function in the array of questions and answers. I would just leave the questions and answers themselves in the array and call the prompt function inside the playerTotals function, something like this:
I've also put the call to
playerResults()
inside theplayerTotals
function, so now you only need to call one function to run the quiz. You could even make the variablescorrect
andincorrect
local variables declared inside theplayerTotals
function, and then callplayerResults(correct, incorrect)
.What you have is good and should work well, I'm just offering a few ideas!