Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial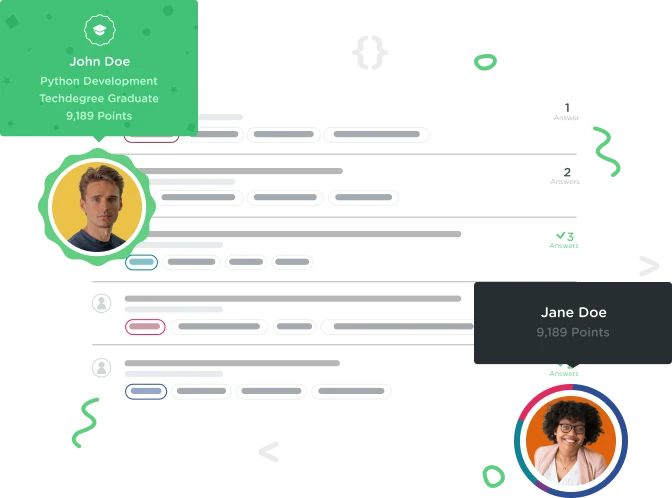

Joel Greek
4,421 PointsHere is a solution for removing specific list Item.
I thought it would be fun (and make more sense for a real project) to create for every li that can remove the specific item created, instead of just the last item created. Here is my solution, let me know what you think!
Using the same css as everyone else so just posting the Javascript and HTML. Notice that I deleted the tasks/items/li-elements from the course (for no other reason then that I didn't want them there.). I am also using different names for the variables and the class names.
Javascript:
const descriptionInput = document.querySelector('input.description');
const descriptionP = document.querySelector('p.description');
const descriptionButton = document.querySelector('button.description');
const toggleList = document.querySelector('button.toggleList');
const listDiv = document.querySelector('.list');
const addItemInput = document.querySelector('input.addItemInput');
const addItemButton = document.querySelector('button.addItemButton');
const removeItemButton = document.querySelector('button.removeItemButton');
toggleList.addEventListener('click', () => {
if (listDiv.style.display == 'none') {
toggleList.textContent = 'Hide List';
listDiv.style.display = 'block';
} else {
toggleList.textContent = 'Show List';
listDiv.style.display = 'none';
}
});
descriptionButton.addEventListener('click', () => {
descriptionP.innerHTML = descriptionInput.value + ':';
descriptionInput.value = '';
});
addItemButton.addEventListener('click', () => {
let ulItemList = document.getElementsByClassName('ulItemList')[0];
let newItem = document.createElement('li');
newItem.textContent = addItemInput.value;
ulItemList.appendChild(newItem);
addItemInput.value = '';
let deleteItem = document.createElement('button');
deleteItem.style.marginLeft = '10px';
deleteItem.textContent = 'X';
deleteItem.addEventListener('click', () => {
newItem.parentNode.removeChild(newItem);
});
newItem.appendChild(deleteItem);
});
html:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<p>Making a web page interactive</p>
<button class="toggleList">Hide List</button>
<div class="list">
<p class="description">Things that are purple:</p>
<input type="text" class="description" />
<button class="description">Change list description</button>
<ul class="ulItemList"></ul>
<input type="text" class="addItemInput" />
<button class="addItemButton">Add list item</button>
</div>
<script src="app.js"></script>
</body>
</html>
1 Answer
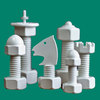
Steven Parker
229,732 PointsGood job. But you're getting a bit ahead of things. A later course exercise will have you build a list where each item will have a "remove" button on it.
musab abdi
7,110 Pointsmusab abdi
7,110 Pointssolve this https://w.trhou.se/7000qz679f . i want to remove the last item on the list . tell me what is wrong with my code. this is the error am getting when i clicked remove button;
app.js:14 Uncaught DOMException: Failed to execute 'removeChild' on 'Node': The node to be removed is not a child of this node. at HTMLButtonElement.<anonymous> (http://port-80-mzfpq0h79b.treehouse-app.com/app.js:14:18)
Steven Parker
229,732 PointsSteven Parker
229,732 PointsStart a fresh question and post your snapshot there. Also provide a link to the course page you are working with.