Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial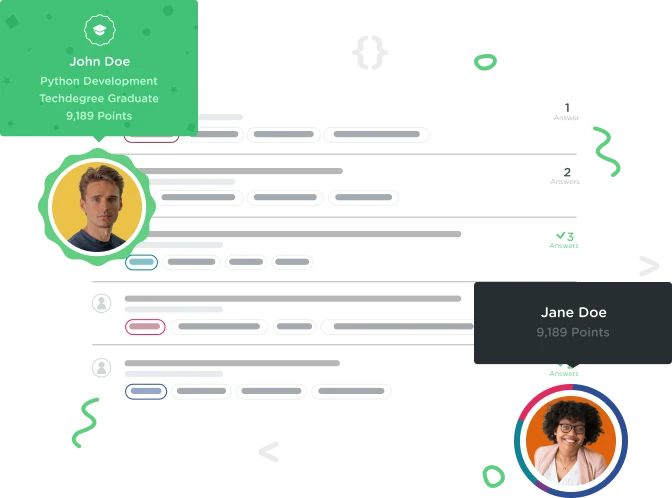

Craig Sweaton
6,386 PointsHere is how I completed the challenge (with a couple of enhancements)
I have added a piece of code that will add the word 'a' or 'an' to the string depending on whether or not the adjective begins with a consonant or vowel.
// The GetPrompt() function will accept a question, display a prompt with that question, then return the answer.
function GetPrompt(question) {
return prompt(question).toLowerCase();
}
// The CheckIfAdjectiveIsVowel() function will insert an 'a' or 'an' into the madlib depending on whether the adjective starts with a vowel or consonant.
function CheckIfAdjectiveIsVowel(adjective) {
switch(adjective.charAt(0)) {
case 'a':
case 'e':
case 'i':
case 'o':
case 'u':
return 'an';
break;
default:
return 'a';
break;
}
}
// We assign the values returned by the get prompt function to the variables.
var adjective = GetPrompt('First, give me an adjective');
var verb = GetPrompt('Next, how about a verb?');
var noun = GetPrompt('Finally, let\'s have a noun');
// We build our madlib string.
var madLib = 'There was once ' + CheckIfAdjectiveIsVowel(adjective) + ' ' + adjective + ' programmer who learned JavaScript to ' + verb + ' the ' + noun + '.';
// We write the madlib string to the page.
document.write(madLib);
2 Answers
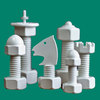
Steven Parker
231,269 PointsCute idea. But why not include the word in the return to make the call more compact:
var addArticle = adjective => ('aeiou'.includes(adjective[0]) ? 'an ' : 'a ') + adjective;
// so later on when you call the method you don't also need to add the word ...
var madLib = 'There was once ' + addArticle(adjective) + ' programmer who learned JavaScript to '
+ verb + ' the ' + noun + '.';
Note: I also rewrote the function for compactness but it's not nearly as efficient.
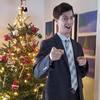
Andrew Hickman
Full Stack JavaScript Techdegree Student 10,013 PointsThat's a great use of a switch statement. I personally despise switch statements but here it makes sense to use it. Cool!
Andrew Hickman
Full Stack JavaScript Techdegree Student 10,013 PointsAndrew Hickman
Full Stack JavaScript Techdegree Student 10,013 PointsWow. Neat!
Craig Sweaton
6,386 PointsCraig Sweaton
6,386 PointsNice solution. You could even use a template string to make the madLib assignment shorter.
var madLib = `There was once ${addArticle(adjective)} programmer who learned JavaScript to ${verb} the ${noun}.`;
Steven Parker
231,269 PointsSteven Parker
231,269 PointsI almost did .. but I felt I was getting carried away enough as it was.