Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial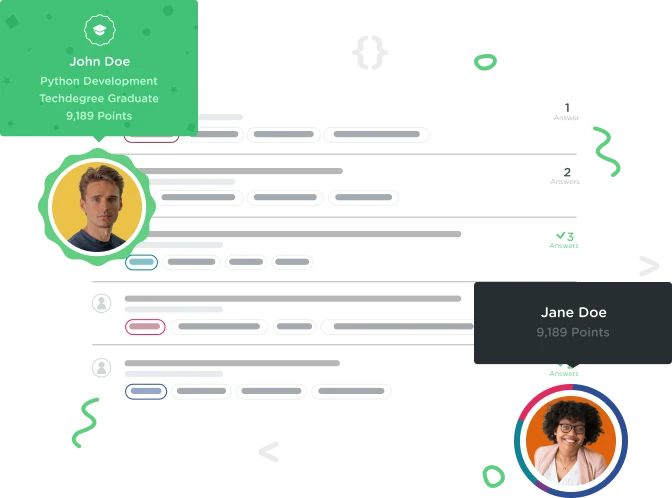

HAROLD MORI
3,629 PointsHere is my approach!
Hope it can help you guys
by the other hand, if anybody can do it in a better way I'm totally open to receive all commets, we are here for learning.
cheers.
player_name = []
answer_player_name = input("Would you like to add a player? Y/N ").lower()
if answer_player_name == "y":
while answer_player_name == "y":
new_name = input( "Please write the name ").title()
player_name.append (new_name)
answer_player_name = input("Would you like to add another player? Y/N ").lower()
print(player_name)
number_of_player = len(player_name)
print("There are {} players on the team.".format(number_of_player))
for x in range (0,len(player_name)):
print("Player ", int(x+1),":" , player_name[x])
num_keeper = int(input("Escoger el número de Jugador. (1 - {}) ".format(number_of_player)))
name_keeper = player_name[num_keeper-1]
print("Great!! the Keeper will be {}!!!".format(name_keeper))
else:
print("OK dude, see you later")
[MOD: added ```python formatting -cf]
1 Answer
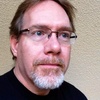
Chris Freeman
Treehouse Moderator 68,441 PointsOverall, the code is good. The following would make the code more readable or quicker to understand. I'm going to be a bit nitpicky, but the goal is always better code. Good on you for putting your code up for review!
- I start by suggesting a bit better formatting to be "Pythonic". You can check standard style using
flake 8
:
# assuming your code is in the file player.py
$ pip install flake8
$ python -m flake8 player.py
player.py:6:1: W293 blank line contains whitespace
player.py:7:3: E111 indentation is not a multiple of four
player.py:7:35: W291 trailing whitespace
player.py:8:1: W293 blank line contains whitespace
player.py:9:22: E201 whitespace after '('
player.py:10:1: W293 blank line contains whitespace
player.py:11:23: E211 whitespace before '('
player.py:11:34: W291 trailing whitespace
player.py:12:1: W293 blank line contains whitespace
player.py:13:23: E221 multiple spaces before operator
player.py:13:80: E501 line too long (87 > 79 characters)
player.py:14:1: W293 blank line contains whitespace
player.py:16:3: E303 too many blank lines (2)
player.py:16:3: E111 indentation is not a multiple of four
player.py:17:1: W293 blank line contains whitespace
player.py:18:3: E111 indentation is not a multiple of four
player.py:19:1: W293 blank line contains whitespace
player.py:20:3: E111 indentation is not a multiple of four
player.py:21:1: W293 blank line contains whitespace
player.py:22:3: E111 indentation is not a multiple of four
player.py:22:17: E211 whitespace before '('
player.py:22:20: E231 missing whitespace after ','
player.py:23:1: W293 blank line contains whitespace
player.py:24:30: E231 missing whitespace after ','
player.py:24:34: E203 whitespace before ','
player.py:25:1: W293 blank line contains whitespace
player.py:26:3: E111 indentation is not a multiple of four
player.py:26:13: E221 multiple spaces before operator
player.py:26:80: E501 line too long (95 > 79 characters)
player.py:27:1: W293 blank line contains whitespace
player.py:28:3: E111 indentation is not a multiple of four
player.py:29:1: W293 blank line contains whitespace
player.py:30:3: E111 indentation is not a multiple of four
player.py:31:1: W293 blank line contains whitespace
player.py:33:1: W293 blank line contains whitespace
player.py:34:3: E111 indentation is not a multiple of four
To auto-correct into hard compliance, you can use the module black
:
$pip install black
$ python -m black player.py
reformatted player.py
All done! ✨ 🍰 ✨
1 file reformatted.
results:
player_name = []
answer_player_name = input("Would you like to add a player? Y/N ").lower()
if answer_player_name == "y":
while answer_player_name == "y":
new_name = input("Please write the name ").title()
player_name.append(new_name)
answer_player_name = input(
"Would you like to add another player? Y/N "
).lower()
print(player_name)
number_of_player = len(player_name)
print("There are {} players on the team.".format(number_of_player))
for x in range(0, len(player_name)):
print("Player ", int(x + 1), ":", player_name[x])
num_keeper = int(
input("Escoger el número de Jugador. (1 - {}) ".format(number_of_player))
)
name_keeper = player_name[num_keeper - 1]
print("Great!! the Keeper will be {}!!!".format(name_keeper))
else:
print("OK dude, see you later")
The still leaves a line over 79 characters:
$ python -m flake8 player.py
player.py:28:80: E501 line too long (82 > 79 characters)
This can be fixed using f-string
formatting:
num_keeper = int(
input(f"Escoger el número de Jugador. (1 - {number_of_player}) ")
)
Finally, my preference would be avoid double spacing every line. Instead, use a blank line to divide sections:
player_name = []
answer_player_name = input("Would you like to add a player? Y/N ").lower()
if answer_player_name == "y":
while answer_player_name == "y":
new_name = input("Please write the name ").title()
player_name.append(new_name)
answer_player_name = input(
"Would you like to add another player? Y/N "
).lower()
print(player_name)
number_of_player = len(player_name)
print("There are {} players on the team.".format(number_of_player))
for x in range(0, len(player_name)):
print("Player ", int(x + 1), ":", player_name[x])
num_keeper = int(
input("Escoger el número de Jugador. (1 - {}) ".format(number_of_player))
)
name_keeper = player_name[num_keeper - 1]
print("Great!! the Keeper will be {}!!!".format(name_keeper))
else:
print("OK dude, see you later")
- Variable name choices can help clarify the code.
For example, answer_add_player
might be a better choice instead of answer_player_name
since there isn't a name involved yet, only the decision to add a player or not.
- Improving the for loop, the code goes through extra work to create an enumerated list. Instead use
enumerate
:
# this
for x in range (0,len(player_name)):
print("Player ", int(x+1),":" , player_name[x])
# becomes
for idx, name in enumerate(player_name, start=1):
print("Player ", idx, ":", name)
# or using f-strings:
# print(f"Player {idx}: {name}")
The code int(x+1)
could simply be (x + 1)
since both are int
s already.
Most of these critiques come from reading lots of code. They will come naturally as you read more code of others (highly recommend). Good luck!!
HAROLD MORI
3,629 PointsHAROLD MORI
3,629 PointsGreat, thanks, I'm checking it!