Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial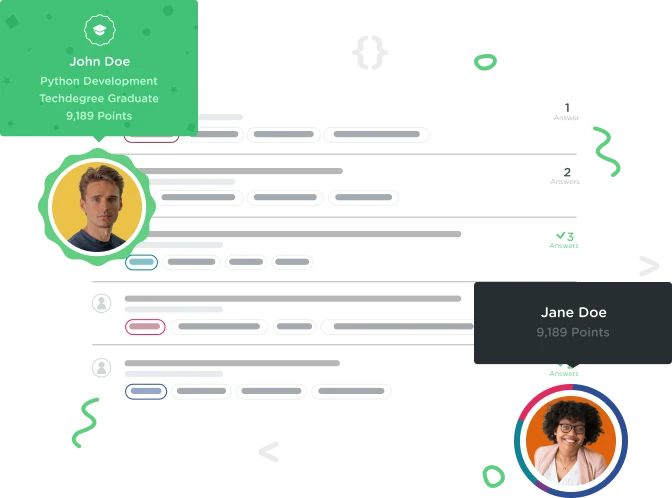

Amy Shah
25,337 PointsHere is my attempt -- what is wrong with this?
I have attempted to follow the directions and I dont think this is working
using Treehouse.Models;
namespace Treehouse.Data
{
public class VideoGamesRepository
{
public VideoGame[] GetVideoGames(int id)
{
return _videoGames(VideoGame)};
}
private static VideoGame[] _videoGames = new VideoGame[]
{
new VideoGame()
{
Id = 1,
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = true
},
new VideoGame()
{
Id = 2,
Title = "Mario Kart 64",
Description = "Mario Kart 64 is a 1996 go-kart racing game developed and published by Nintendo for the Nintendo 64 video game console.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = false
}
};
}
}
namespace Treehouse.Models
{
// Don't make any changes to this class!
public class VideoGame
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public string[] Characters { get; set; }
public string Publisher { get; set; }
public bool Favorite { get; set; }
public string DisplayText
{
get
{
return Title + " (" + Publisher + ")";
}
}
}
}
3 Answers
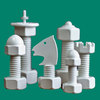
Steven Parker
231,268 PointsYou have a few issues here:
- The challenge said your method "shouldn't accept any parameters", but your method takes an int named "id".
- The challenge said to "return the _videoGames private static field", but your return seems to be calling a method with that name.
- You also have a stray close brace ("
}
") at the end of the return statement.

Amy Shah
25,337 PointsI solved this one. Thanks everyone. There few questions were the ones I did not know how to do. Thanks

Henrique Vignon
Courses Plus Student 6,415 Pointsedit:: ugggh, nvm, You actually have to return the instance in the array which has the ID that corresponds to the argument, in that case you'll need to run foreach loop to find it, example:
foreach (VideoGame videoGame in _videoGames)
{
if (videoGame."property you want to check" == "argument")
{
return videoGame;
}
}

Henrique Vignon
Courses Plus Student 6,415 PointsAlso you seem to be having a bit of a problem with some core aspects of the language, I highly recommend you go trough the C# basics, C# Collections and C# objects (and perhaps intermediate C# as well) courses before you tackle ASP development.
Henrique Vignon
Courses Plus Student 6,415 PointsHenrique Vignon
Courses Plus Student 6,415 Pointsnah she's at the second phase of that challenge
Steven Parker
231,268 PointsSteven Parker
231,268 PointsEach task should create a separate method.
Creating GetVideoGames (plural) is task 1. Creating GetVideoGame (singular) is task 2. The task 1 code must remain as-is when task 2 is added.
Perhaps the whole issue is a result of confusing the task 2 instructions and trying to mix the two separate methods into one?
Henrique Vignon
Courses Plus Student 6,415 PointsHenrique Vignon
Courses Plus Student 6,415 PointsOh! you're absolutely right, it has to be a different method, one gets the entire array of videogames, and the other gets the particular instance that has the Id specified