Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial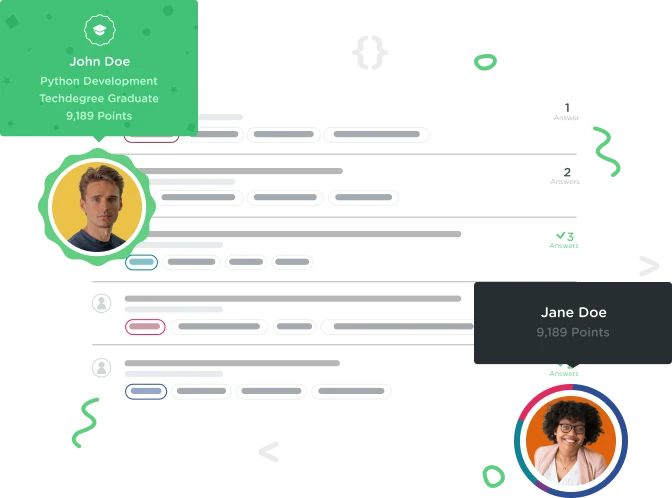
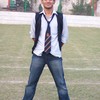
Shivang Chaturvedi
2,600 PointsHere is my code
var num1 = parseInt(prompt("Enter the 1st Number"));
var num2 = parseInt(prompt("Enter the 2nd Number"));
function randomNumber(a, b)
{
if(a > b)
{
var randomNum = Math.floor(Math.random() * (a - b + 1)) + b;
alert(randomNum);
}
else
{
var randomNum = Math.floor(Math.random() * (b - a + 1)) + a;
alert(randomNum);
}
}
var numArgument = randomNumber(num1, num2);
Would love to know if anyone has a better way to do it.
4 Answers
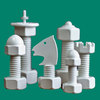
Steven Parker
231,269 PointsHere's a possible alternative:
function randomNumber(a, b) {
if (a > b) [a, b] = [b, a];
return Math.floor(Math.random() * (b - a + 1)) + a;
}
var numArgument = randomNumber(
parseInt(prompt("Enter the 1st Number")),
parseInt(prompt("Enter the 2nd Number"))
);
alert(numArgument);
The argument swapping shown on the 2nd line makes use of a technique called destructuring assignment.
Also note that in the original code, randomNumber did not return anything, so the assignment of numArgument would always be "undefined
". But if you add "return randomNum;
" at the end of the function then operationally it will be identical to this code.
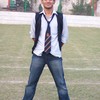
Shivang Chaturvedi
2,600 PointsHey Steven Parker .. I understood what it is doing!! Thanks..
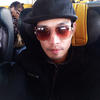
rayjens code
882 Pointshello. i cant understand, because it says generate random number not input number right? or i misunderstand the question? my mind is flying away the topic is starting to be harder >.<
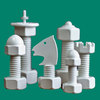
Steven Parker
231,269 PointsThe input is for the range of the random number. The formula picks a random number so it will never be less than the low number you give, and never higher than the high number you give. So for example, if you were to input the numbers 20 and 30, you're asking the computer to "pick any number between 20 and 30".
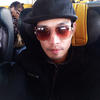
rayjens code
882 Pointsahh i see . thanks for that bro. I feel harder to understand the topics, maybe the lecturer at this stage is so fast >.<
Shivang Chaturvedi
2,600 PointsShivang Chaturvedi
2,600 PointsSteven Parker .. Thank You for sharing this new technique. Its a wonderful piece of code. Can you please tell me more about this block:
if (a > b) [a, b] = [b, a];
Its not getting around my head very clearly. What is [a, b] = [b, a] doing?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThat's a destructuring assignment. As used here, it's just a quick way of swapping two values.