Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial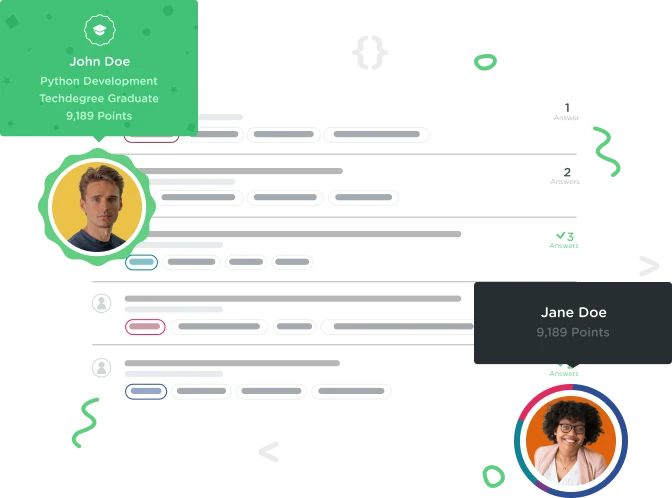

Paul Adams
11,105 PointsHere is my code for the extended challenge for the JavaScript Loops, Arrays and Objects course.
I'm fairly new to JavaScript and programming, if anyone could give feedback on my code, I'd be very grateful.
I have also included a flag variable to break the loop once records have been found, so you don't have to type 'quit' or select 'Cancel' for the programme to print the results.
// Set Global Variables
let message = '';
let student;
let studentList = [];
let search;
let flag = true;
/**
* Prints out supplied html to page
* @param {string} message - Content to be printed to the website
*/
function print (message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
/**
* Loop through an array of objects to create student details
* @param {Array,<object>} studentList - An array of student objects
* @returns {String} returns the html markup to be printed to the website
*/
function getStudentReport (studentList) {
let report ='';
for(let i = 0; i< studentList.length; i++) {
report += `<h2>Student: ${studentList[i].name}</h2>`;
report += `<p>Track: ${studentList[i].track}</p>`;
report += `<p>Points: ${studentList[i].points}</p>`;
report += `<p>Achievements: ${studentList[i].achievements}</p>`;
}
return report;
}
// While loop to display prompt to search student records
while (flag) {
search = prompt( 'Search for a student by entering a name. Type quit to exit');
// If user selects Cancel, or user types quit - exit the loop
if (search === null || search.toLowerCase() === 'quit') {
break;
}
// For loop to see if name provided matches a student(s) name
for (var i = 0; i < students.length; i ++) {
student = students[i];
if (student.name === search) {
studentList.push(student);
}
}
// If name does not match a student(s) name - Display an alert
if (studentList.length === 0) {
alert(`Sorry, there are no students called ${search}`);
}
/* If matches, send records to the getStudentReport function,
print message and set flag to false and break loop
*/
else if (studentList.length > 0) {
message = getStudentReport(studentList);
print(message);
flag = false;
}
}
1 Answer
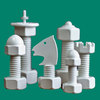
Steven Parker
231,269 PointsInteresting choice, so you sacrificed the ability to look up more than one name to see a single one immediately.
But don't worry, you'll soon learn other methods that will allow you to achieve both objectives at the same time.
Paul Adams
11,105 PointsPaul Adams
11,105 PointsThanks for your comment Steven. As you say, I currently do not know another way to gracefully achieve this. I just wanted to display the results once a record(s) have been found.
Hopefully soon I will be able to review this project and have a better solution. I know I have a long, and sometimes frustrating path ahead of me XD.
Many thanks :)