Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial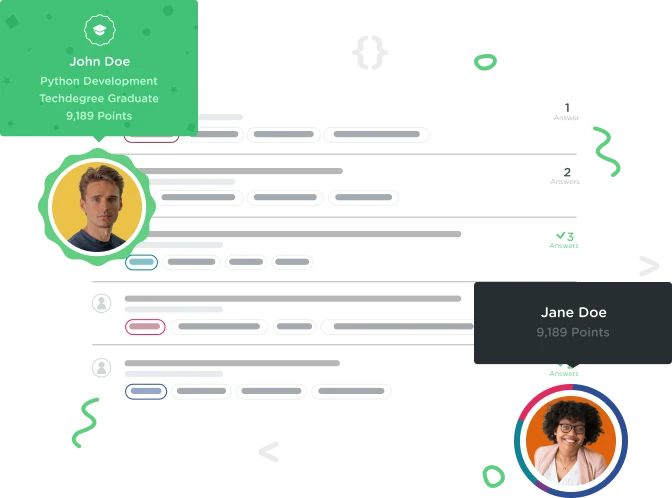

rajeves
14,300 PointsHere is my extended version of the dungeon game.
import os
import random
def clear_screen():
os.system('cls' if os.name == 'nt' else 'clear')
def get_locations(CELLS):
return random.sample(CELLS, 4) #does not include repeats
def move_player(player, move):
x, y = player
if move == "LEFT":
x -= 1
if move == "RIGHT":
x += 1
if move == "UP":
y -= 1
if move == "DOWN":
y += 1
return x, y
def get_moves(player, play_field):
moves = ["LEFT", "RIGHT", "UP", "DOWN"]
x, y = player
if x == 0:
moves.remove("LEFT")
if x == play_field - 1:
moves.remove("RIGHT")
if y == 0:
moves.remove("UP")
if y == play_field - 1:
moves.remove("DOWN")
return moves
def draw_map(player, path, CELLS, play_field):
print(" _"*play_field)
tile = "|{}"
for cell in CELLS:
x, y = cell
if x < play_field - 1:
line_end = ""
if cell == player:
output = tile.format("X")
elif cell in path:
output = tile.format("*")
else:
output = tile.format("_")
else:
line_end = "\n"
if cell == player:
output = tile.format("X|")
elif cell in path:
output = tile.format("*|")
else:
output = tile.format("_|")
print(output, end=line_end)
def draw_cheat_map(player, path, CELLS, play_field, door, monster1, monster2):
print(" _"*play_field)
tile = "|{}"
for cell in CELLS:
x, y = cell
if x < play_field - 1:
line_end = ""
if cell == player:
output = tile.format("X")
elif cell == monster1:
output = tile.format("M")
elif cell == monster2:
output = tile.format("M")
elif cell == door:
output = tile.format("D")
elif cell in path:
output = tile.format("*")
else:
output = tile.format("_")
else:
line_end = "\n"
if cell == player:
output = tile.format("X|")
elif cell == monster1:
output = tile.format("M|")
elif cell == monster2:
output = tile.format("M|")
elif cell == door:
output = tile.format("D|")
elif cell in path:
output = tile.format("*|")
else:
output = tile.format("_|")
print(output, end=line_end)
def game_loop():
while True:
try:
CELLS = []
play_field = int(input("Enter a number for size of playing field 2 or greater: "))
if play_field >= 2:
for y in range(play_field):
for x in range(play_field):
CELLS.append((x, y))
monster1, monster2, door, player = get_locations(CELLS)
playing = True
path = []
score = 0
break
else:
print("The playing field must be 2 or greater!")
except ValueError:
print("Please enter numeric values only!")
while playing:
clear_screen()
draw_map(player, path, CELLS, play_field)
valid_moves = get_moves(player, play_field)
print("You're currently in room {}".format(player))
print("Current score: {} PTS".format(score))
print("You can move {}".format(", ".join(valid_moves)))
print("Enter QUIT to quit or CHEAT to cheat")
path.append(player)
score += 5
move = input("> ").upper()
if move == "QUIT":
print("\n ** See you next time ** \n")
break
elif move == "CHEAT":
clear_screen()
draw_cheat_map(player, path, CELLS, play_field, door, monster1, monster2)
print("Icon key: X = Player; M = Monsters; D = Door.")
input("Press Enter to stop cheating. ")
continue
if move in valid_moves:
player = move_player(player, move)
if player == monster1 or player == monster2:
print("\n ** oh no! The monster got you! Better luck next time! **\n")
playing = False
if player == door:
print("\n ** You escaped! Congratulations! **\n")
playing = False
else:
input("\n ** Walls are hard! Don't run into them! **\n")
continue
else:
if input("Play again? [Y/n] ").lower() != "n":
game_loop()
clear_screen()
print("Welcome to the dungeon!")
input("Press Enter to start game! ")
clear_screen()
game_loop()
1 Answer

Nataly Rifold
12,432 PointsI have 2 questions:
- why do a for loop? and how come python knows that the same input can be used as x and y? this code is suppose to create the CELL list as I understand. but can't you just put range instead of int (range gives you a list)? and after that the CELL.append?
play_field = int(input("Enter a number for size of playing field 2 or greater: ")) if play_field >= 2: for y in range(play_field): for x in range(play_field): CELLS.append((x, y))
- I can't understand the coda: if x == play_field - 1:
you use the play_field to set the map size, so if it 2, then that's the end of the map. why 2 - 1? if its index isn't supposed to be in []?
Milad Latif
6,189 PointsMilad Latif
6,189 PointsNice! I like how you get points for exploring and also that it shows where you have already been with the *.