Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial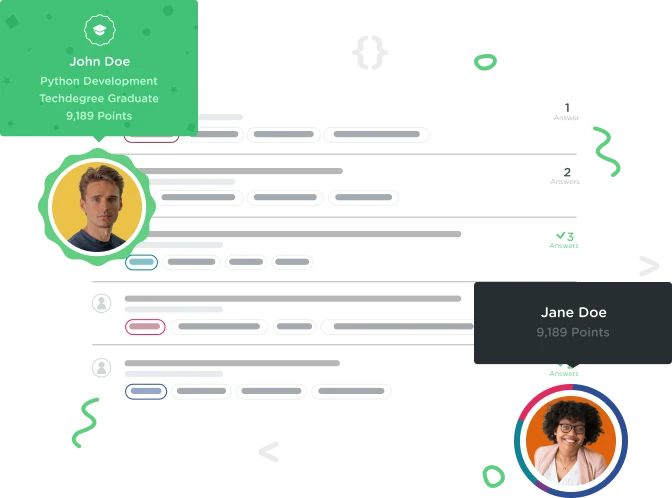

fahad lashari
7,693 PointsHere is my number guessing game reverse engineered for the computer
Hi everyone, at the end of the last video kenneth suggested it would be good practice to reverse engineer the number guessing game so that I pick the number and the computer tries to guess.
I took this a litte further by allowing the user an option to give the computer a HINT, if the uses choose yes then they may pick between the hints "guess higher" or "guess lower".
The computer will then guess higher in comparison to their last guess.
Once the computer is out of tries it will end the game and ask to play again.
Hope you guys enjoy it and can provide some feedback.
import random
#generate a number between 1 and 10
def show_help():
print("""Welcome! Let's play a guessing game. Enter a number for me to guess. Type "HELP" to see all
options
Type "END" to end the game
Type "RESTART" to start the game again from the beginning
Goodluck!
""")
def game_if_hint_Y(sec_number, guess):
hint = input("""Computer says: THANK YOU!!
To provide a hint say "guess higher" or "guess lower" """)
tries = 3
while True:
tries -= 1
if hint.upper() == "GUESS HIGHER":
if tries <= 0:
print("""Computer says: I am out of tries! :'( """)
break
else:
print("""Computer says: I have {} tries left""".format(tries))
print("Computer says: ok great. let me guess higher")
computer_guess = random.randint(guess, 10)
print("Computer says: hmm, my guess is {}".format(computer_guess))
if computer_guess == sec_number:
print("""Computer says: Finally! that was tough!
Good playing with you""")
break
else:
print("""Computer says: let me try again""")
elif hint.upper() == "GUESS LOWER":
if tries <= 0:
print("""Computer says: I am out of tries! :'( """)
break
else:
print("""Computer says: I have {} tries left""".format(tries))
print("Computer says: alright, let me guess abit lower")
computer_guess = random.randint(1, guess)
print("Computer: I guess {}".format(computer_guess))
if computer_guess == sec_number:
print("""Computer says: Finally! that was tough!
Good playing with you""")
break
else:
print("""Computer says: let me try again""")
def game():
show_help()
counter = 0
tries = 5
hint_needed_counter = 3
secret_number = int(input("""Computer says: Please type a number between 1-10 for me to guess\n """))
while True:
counter += 1
tries -= 1
hint_needed_counter -= 1
#get a number guess from the player
computer_guess = random.randint(1, 10)
#compare guess to secret number
if computer_guess == secret_number:
#print hit/miss
print("""Computer says: I guess {}!""".format(computer_guess))
print("""Computer says: I got it right!""")
print("""Computer says: It took me {} trie(s)""".format(counter))
print("""Computer says: Good game. let's play again sometime""")
break
elif counter >= 5:
print("""Computer says: I am out of tries! :'( """)
break
elif hint_needed_counter == 0:
provide_hint = input("""Computer says: this is pretty difficult, Can I have a hint? :)
Y/N """)
if provide_hint.upper() == "Y":
game_if_hint_Y(secret_number, computer_guess)
break
else:
print("I guess {}!".format(computer_guess))
print("Whoops! got it wrong. let me try again: ")
print("Tries left: {}".format(tries))
#Provide the player with a hint
#provide_hint = input("Computer says: can you help me out with a hint")
play_again = input("Would you like to play the game again? Y/n")
if play_again.lower() != 'n':
game()
game()
Enjoy!