Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial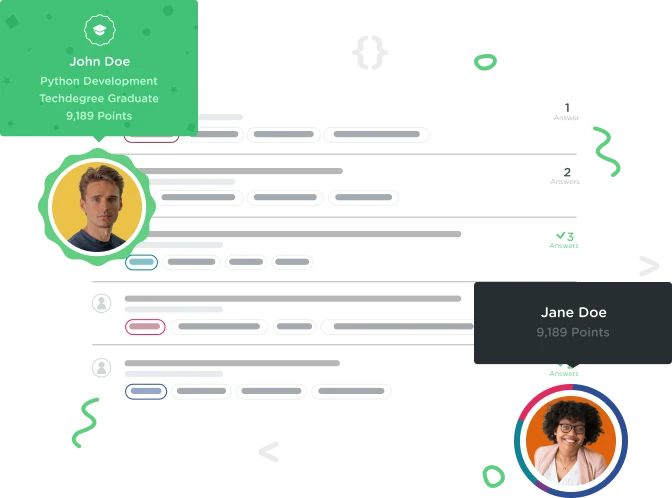

fahad lashari
7,693 PointsHere is my own solution to this problem with least possible loops and if statements
I could be entirely wrong but I think using while loops too often along with if statement might not be the most efficient as the programme loops through and runs every statement even if it did not need to be run. I have taken a slightly different approach. Here the functions only run if and when the user requires them to run. I'd appreciate any feedback. If this code is painful to read I apologise in advance. I have only just completed the python basics course. Also I have not yet implemented any functionality that stops the user from type incorrect Values e.g. if you type in a String where the programme asks for a index value, the programme will just throw an error, I will be adding this later. Also I have not added the functionality to added multiple items at once as I forgot, you can add it in as you wish.
EDIT: just added the functionality of adding more than one item with a loop. Still can't figure out how to implement a 'strip()' function without using a loop. If you know, do let me know.
Please test it out in your workspaces and let me know what you think
Kind regards
Fahad
import sys
shopping_list = []
command = input("""Would you like to start your supreme AI shopping experience?\n Y/n""")
def show_help():
print('\nSeperate each item with a comma.')
print('\nType DONE to quit, SHOW to see the current list, and HELP to get this message')
add_item()
def show_list():
count = 1
for item in shopping_list:
print('{}: {}'.format(count, item))
count+=1
add_item()
def add_item():
command = input('\nPlease enter the name of the item:\nSeperate each item with a comma if more than one item\n')
if command != "DONE".lower() and command != "SHOW".lower() and command != "HELP".lower():
index = input("Add this to certain spot on the list?, "
"\ncurrently we are on number {}\nPRESS ENTER for last spot and continue or give me a NUMBER. NO LETTERS or " "SYMBOLS:\n".format(len(shopping_list)))
if "," in command:
shopping_list.extend((command.split(',')))
if index:
shopping_list.insert((int(index)-1), command.strip())
elif not "," in command:
shopping_list.append(command.strip())
add_item()
else:
function_dict[command]()
def exit():
print("""Thank for shopping with us.
I know it's a bit late but we dont really sell anything...\nThe shopping list looks neat though!\nOk bye now""")
sys.exit()
function_dict = {"Y".lower():add_item, "HELP".lower():show_help, "SHOW".lower():show_list, "DONE".lower():exit}
function_dict[command]()
1 Answer

Beshoy Megalaa
3,429 PointsYour assumption of the usage of while loop being inefficient is incorrect. Conditional statements such as if and else and keywords such as break and continue stops the while loop from running every. For example, if the user input == 'DONE' the the while loop ends because of the break statement despite of its setup to run forever. Therefore, only few lines ran and rest ignored since loop terminated.
Your approach to use recursion (the function calling itself) works, but it's more expensive(trivial in this case) in terms of memory resources and not easy to understand. In my opinion, the while loop is more elegant and simpler to understand the logics.
fahad lashari
7,693 Pointsfahad lashari
7,693 PointsHi Beshoy thanks for responding. I am just wondering, why is this approach more expensive on the memory? I understand it may not be easy to understand (which is definitely a negative side). However I would still like to know why this approach vs while and a chain of if else statements are more efficient?