Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial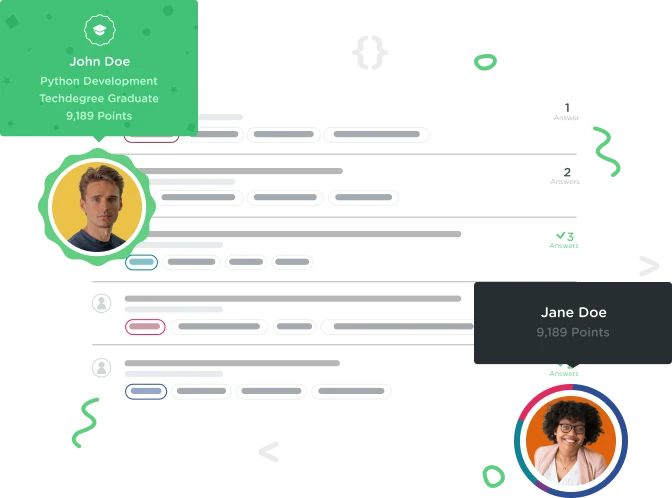

fahad lashari
7,693 PointsHere is my refinement of the number game:
*The player is told to guess a number between 1 and 10 *The player is then notified whether the guess is correct or not if not, then the player is provided with a hint as to whether they should guess higher or *lower. They are also informed about the number of tries they have left *once the player guess the right answer or runs out of tries. The game ends
import random
#generate a number between 1 and 10
secret_number = random.randint(1, 10)
counter = 0
tries = 5
while True:
counter += 1
tries -= 1
#get a number guess from the player
player_guess = int(input("""I am thinking of a number between 1 and 10
Take a guess: """))
#compare guess to secret number
if player_guess == secret_number:
#print hit/miss
print("""Congrats! you got it right!""")
break
elif counter == 5:
print("""No more tries left!""")
break
else:
print("Whoops! you got it wrong. Try again: ")
print("Tries left: {}".format(tries))
#Provide the player with a hint
if player_guess < secret_number:
print("HINT: guess higher")
elif player_guess > secret_number:
print("HINT: guess lower")
PYTHON
Hope I have helped some one
Kind regards

fahad lashari
7,693 PointsYou're welcome :D do let me know if you need anymore help
2 Answers
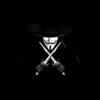
Sky Lu
1,260 PointsI got an easy way to make it.
import random
# generate a random number between 1 and 10
secret_num = random.randint(1, 10)
# Player only got 5 chances to guess the number
chances = 5
while True:
chances -= 1
# get a number guess from the palyer
guess = int(input("Give me a number between 1 and 10: "))
# compare guest to the secret number
if guess == secret_num:
print("Wow, u got the number {}!".format(secret_num))
break
# After player done 5 times, quit the game
elif chances == 0:
print("Game Over Baby")
break
elif guess > secret_num:
print("""
Make it smaller
Left {} times to try""".format(chances))
elif guess < secret_num:
print("""
Make it bigger
Left {} times to try""".format(chances))
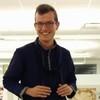
slavster
1,551 PointsNice job guys! Looks like we had the same thought on higher/lower. I also wanted to practice defining an function and calling it multiple times to save on typing. So I added another print line letting the player know what numbers they have already tried :)
import random
tracker = []
def track_input():
print("You already tried {}.".format(tracker))
#generate random number 1-10
secret_num = random.randint(1, 10)
while True:
#get a number guess from player
guess = int(input("Guess a number between 1 and 10: "))
tracker.append(guess)
#compare guess to secret number
if guess == secret_num:
print("You got it! The number was {}".format(secret_num))
break
elif guess < secret_num:
print("Nope! Try higher...")
track_input()
else:
print("Sorry, try lower...")
track_input()
#print hit/miss
Ryan Munsch
6,552 PointsRyan Munsch
6,552 PointsFahad,
Thank you for this!