Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial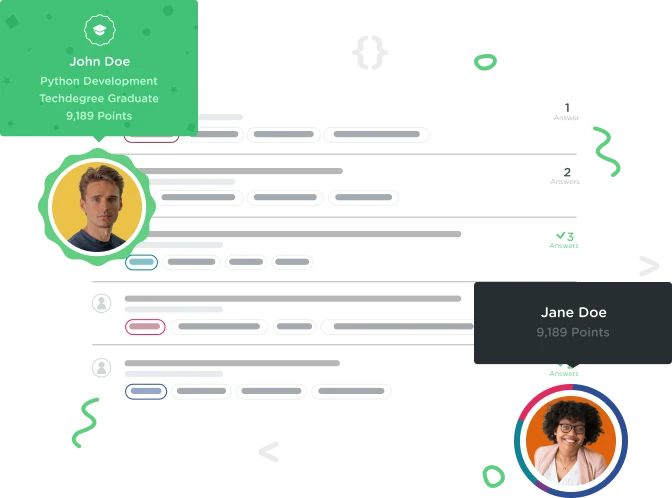
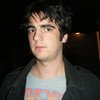
Warren Fairbairn
2,516 PointsHere is my Reverse Number game for Python
It works quite nicely, any tips on how to clean it up or work better would be greatly appreciated thanks.
import random
def secret_number():
while True:
try:
num = int(input("Please enter a number between 1 - 10: "))
except ValueError:
print("That's not a number! Try again!")
continue
if num <1 or num > 10:
print("Invalid range. Number must be between 1 - 10!")
continue
else:
return num
break
def play_again():
replay = input("Do you want to play again? Y/n: ")
if replay == "Y".lower():
game()
def computer_guess():
min = 1
max = 10
guesses = []
while len(guesses) < 5:
input("Press 'Enter' so I can guess a number...\n")
pcguess = random.randint(min, max)
if pcguess in guesses:
pcguess = random.randint(min, max)
else:
guesses.append(pcguess)
print("I guessed {}. Is this correct?".format(pcguess))
print("""
Enter 'yes' if the guess was correct:
Enter 'higher' if the guess was too low:
Enter 'lower' if the guess was too high:
""")
answer = input(" >")
if answer == "yes":
print("Woah! I read your mind!")
break
elif answer == "higher":
min = pcguess
print("I was wrong? Ok I'll guess a higher number!/n")
pcguess = random.randint(min, max)
elif answer == "lower":
max = pcguess
print("I was wrong? Ok I'll guess a lower number!/n")
pcguess = random.randint(min, max)
else:
print("Oh I'm out of guesses? I'll get it next time!")
def game():
secret_num = secret_number()
computer_guess()
play_again()
game()
1 Answer
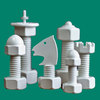
Steven Parker
231,060 PointsA couple of quick comments:
Things I noticed at first glance:
- you don't need a
break
after areturn
(no code following areturn
will ever be used) - you don't need an
else
after anif
which diverts the execution flow (such as withcontinue
) -
"Y".lower()
is the same thing as"y"
(or did you mean to write:return.upper() = "Y"
?)