Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial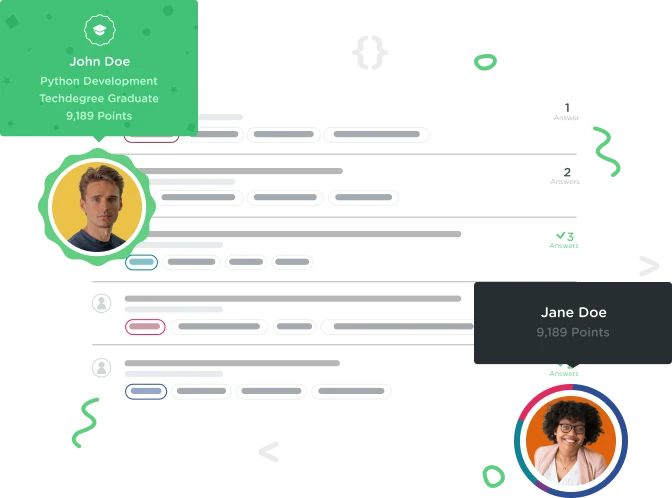

amit dilawri
1,244 PointsHere is my solution
var html = ''; var red; var green; var blue; var rgbColor;
function getRandomColor(){ var randomColor = Math.floor(Math.random() * 256 ); return randomColor; }
function colorMix(){ red = getRandomColor(); green = getRandomColor(); blue = getRandomColor(); return rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')'; }
for (var i = 0; i <= 10; i += 1){ html += '<div style="background-color:' + colorMix() + '"></div>'; }
document.write(html);
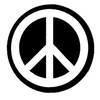
john larson
16,594 PointsI reformatted the code to look better
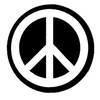
john larson
16,594 PointsLooks Great!!! code looks nice and clean. I forgot to give the div a width and height, so it wasn't showing up. Silly me.
8 Answers
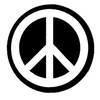
john larson
16,594 Points`

amit dilawri
1,244 PointscolorMix() only works when you call it under var html. html has this div tag which I believe makes the color shows up in the circles.
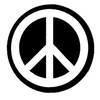
john larson
16,594 PointsI see it now sorry.
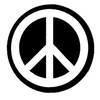
john larson
16,594 PointsIt's not working in my browser. Is it working in your's?

amit dilawri
1,244 PointsJohn Larson - How do you format the code the way you did it?
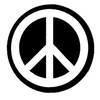
john larson
16,594 Pointsnear the bottom of the page there's a link that tells how to do it. Markdown Cheatsheat. But its: three back tics the word JavaScript. Hit enter a few times. Paste in your code. Hit enter a few more times. Three more back tics, and your done :D

amit dilawri
1,244 PointsHave you copied the css file and index.html from the workspace?

amit dilawri
1,244 Points<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Color Blocks</title>
<link rel="stylesheet" href="styles.css">
</head>
<body id="color">
<script src="script.js"></script>
</body>
</html
@import url('http://necolas.github.io/normalize.css/3.0.2/normalize.css');
/*General*/
body {
background: #fff;
max-width: 980px;
margin: 50px auto;
padding: 0 20px;
font: Helvetica Neue, Helvectica, Arial, serif;
font-weight: 300;
font-size: 1em;
line-height: 1.5em;
color: #8d9aa5;
}
a {
color: #3f8aBf;
text-decoration: none;
}
a:hover {
color: #3079ab;
}
a:visited {
color: #5a6772;
}
h1, h2, h3 {
font-weight: 500;
color: #384047;
}
h1 {
font-size: 1.8em;
margin: 60px 0 40px;
}
h2 {
font-size: 1em;
font-weight: 300;
margin: 0;
padding: 10px 0 30px 0;
}
#home h2 {
margin: -40px 0 0;
}
h3 {
font-size: .9em;
font-weight: 300;
margin: 0;
padding: 0;
}
h3 em {
font-style: normal;
font-weight: 300;
margin: 0 0 10px 5px;
padding: 0;
color: #8d9aa5;
}
ol {
margin: 0 0 20px 32px;
padding: 0;
}
#home ol {
list-style-type: none;
margin: 0 0 40px 0;
}
li {
padding: 8px 0;
display: list-item;
width: 100%;
margin: 0;
counter-increment: step-counter;
}
#home li::before {
content: counter(step-counter);
font-size: .65em;
color: #fff;
font-weight: 300;
padding: 2px 6px;
margin: 0 18px 0 0;
border-radius: 3px;
background:#8d9aa5;
line-height: 1em;
}
.lens {
display: inline-block;
width: 0;
height: 0;
border-top: 8px solid transparent;
border-bottom: 8px solid transparent;
border-right: 8px solid #8d9aa5;
border-radius: 5px;
position: absolute;
margin: 5px 0 0 -19px;
}
#color div {
width: 50px;
height: 50px;
display: inline-block;
border-radius: 50%;
margin: 5px;
}
span {
color: red;
}
var html = '';
var red;
var green;
var blue;
var rgbColor;
function getRandomColor(){
var randomColor = Math.floor(Math.random() * 256 );
return randomColor;
}
function colorMix(){
red = getRandomColor();
green = getRandomColor();
blue = getRandomColor();
return rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
}
for (var i = 0; i <= 10; i += 1){
html = html + '<div style="background-color:' + colorMix() + '"></div>';
}
document.write(html);

amit dilawri
1,244 PointsThanks for your feedback John.
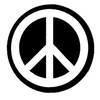
john larson
16,594 Pointslol, you gave me more feedback than I gave you.

amit dilawri
1,244 PointsI got program to work however I do need help in understanding the following lines of codes:
return rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
I don't understand why we have strings such as commas and rgb in the above line of code. Without those strings the code doesn't work.
b) html = html + '<div style="background-color:' + colorMix() + '"></div>';
What code is the div style = background-color referring to? Is it in CSS? If yes, can you explain me where in CSS.
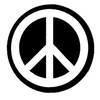
john larson
16,594 Points//this is inline css. we are generally discouraged from doing this
//but in this case I think it's the only way
//it's how they did style before css style sheets
'<div style="background-color:' + colorMix() + '"></div>'
//it would have looked like this
//and would have been on the html page
<h1 style="background-color: red;">This is my Header</h1>
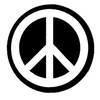
john larson
16,594 Pointsas far as this part
return rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
//this is one of the ways to add color
//an rgb value, it looks like this
//rgb(75, 25, 80);
//so we are generating a string that does the same thing
//as if we had placed the string right in the html

amit dilawri
1,244 PointsGot it. Thank you for your help.
john larson
16,594 Pointsjohn larson
16,594 Points