Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial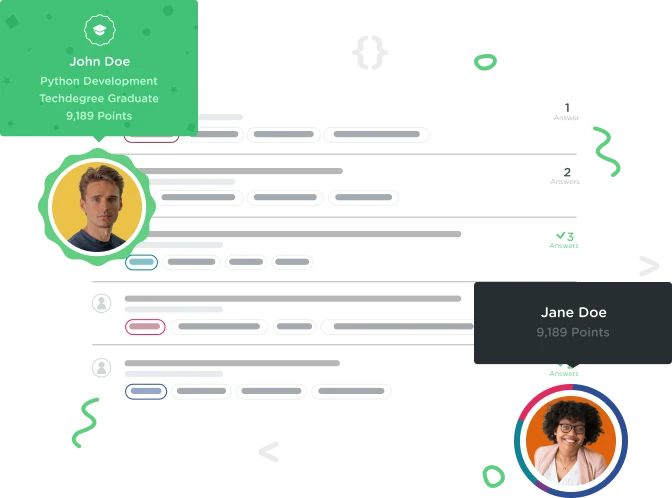
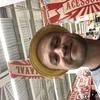
Gennadii Chamorsov
9,207 PointsHere is my solution, everything is working as it should except the part where I used the logical OR operator.
// First question
let correctGuessLondon = false;
let score = 0;
let rank = '';
const ukCapital = prompt('What is the capital of Great Britain?');
if ( ukCapital === 'London') {
correctGuessLondon = true;
}
if (correctGuessLondon === true) {
score = 1;
}
// Second question
let correctGuessTrump = false;
const usPresident = prompt('Who is the president of USA?');
if ( usPresident === 'Donald Trump') {
correctGuessTrump = true;
}
if ( correctGuessTrump === true ) {
score += 1;
}
// Third question
let correctGuessRiver = false;
const bigRiver = prompt('What is the biggest river in Europe?');
if ( bigRiver === 'Danube' ) {
correctGuessRiver = true;
}
if ( correctGuessRiver === true ) {
score += 1;
}
// Forth question
let correctGuessCar = false;
const car = prompt('What is the second word in the Mercedes brand?');
if ( car === 'Benz' ) {
correctGuessCar = true;
}
if ( correctGuessCar === true ) {
score += 1;
}
// Fifth question
let correctGuessFingers = false;
let fingers = prompt('How many fingers has the human?');
if ( fingers === '20' || fingers === 'Twenty' ) /* THAT'S WHERE INPUT "20"
DOESN'T WORK */
{
correctGuessFingers = true;
}
if ( correctGuessFingers === true ) {
score += 1;
}
// Player's rank and Displayed message
if ( score === 0 ) {
rank = 'No Crown';
document.querySelector('main').innerHTML =
`<h1>You got 0 out of 5 questions correct.</h1>
<p>Crown earned: ${rank}</p>`;
} else if ( score === 1 ) {
rank = 'Bronze';
document.querySelector('main').innerHTML =
`<h1>You got 1 out of 5 questions correct.</h1>
<p>Crown earned: ${rank}</p>`;
} else if ( score === 2 ) {
rank = 'Bronze';
document.querySelector('main').innerHTML =
`<h1>You got 2 out of 5 questions correct.</h1>
<p>Crown earned: ${rank}</p>`;
} else if ( score === 3 ) {
rank = 'Silver';
document.querySelector('main').innerHTML =
`<h1>You got 3 out of 5 questions correct.</h1>
<p>Crown earned: ${rank}</p>`;
} else if ( score === 4 ) {
rank = 'Silver';
document.querySelector('main').innerHTML =
`<h1>You got 4 out of 5 questions correct.</h1>
<p>Crown earned: ${rank}</p>`;
} else if ( score === 5 ) {
rank = 'Gold';
document.querySelector('main').innerHTML =
`<h1>You got 5 out of 5 questions correct.</h1>
<p>Crown earned: ${rank}</p>`;
}
1 Answer
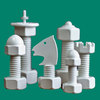
Steven Parker
231,269 PointsThe type-sensitive comparison will never consider a number and a string to match., so the "20" needs to be enclosed in quotes to make it a string.
And when posting code to the forum, use Markdown formatting to preserve the appearance.
Gennadii Chamorsov
9,207 PointsGennadii Chamorsov
9,207 PointsThank you for checking and sorry for the mess, already fixed it. I tried this way:
And still, it doesn't work.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsGennadii Chamorsov — Glad to help. You can mark the question solved by choosing a "best answer".
And happy coding!