Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial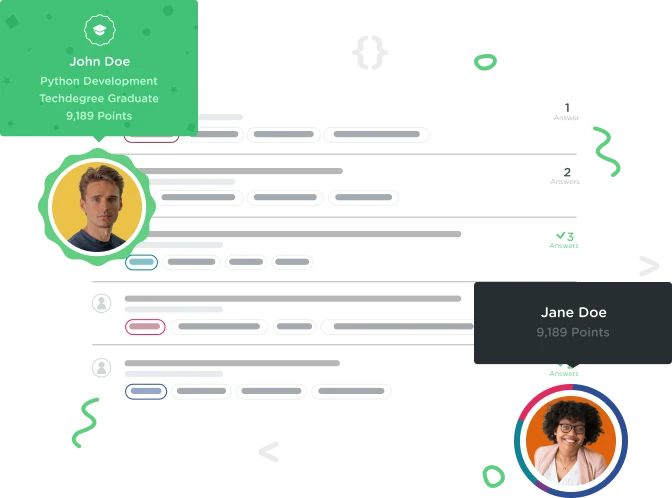

fahad lashari
7,693 PointsHere is my solution for this. What do you guys think?
Also I would really appreciate if someone could just explain to me how I can do this with try and except and I just couldn't figure out how to implement that into my code
words = ["first", "second", "third", "fourth", "fifth"]
words_without_vowels = []
def remove_vowels(word):
new_word=""
non_vowels = []
word = list(word)
for letter in word:
if letter not in "aeiou":
new_word+=letter
words_without_vowels.append(new_word.capitalize())
def return_non_vowel_words():
for word in words:
remove_vowels(word)
print(words_without_vowels)
return_non_vowel_words()
The code works well and does pretty much exactly what's being asked
5 Answers
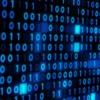
Alexander Davison
65,469 PointsI found an even simpler way using a list comprehension:
def remove_vowels_from(word):
return ''.join([letter for letter in word if letter not in list('aeiou')])
Try it for yourself :P
~Alex
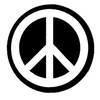
john larson
16,594 PointsHey Alex, list comprehension seems to keep coming up though I don't remember it in the course from Kenneth. It looks like a very shorthand method of other loops and conditionals. Very nice :D I guess I need to look up list comprehension on tutorials point. Thanks.
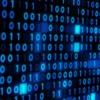
Alexander Davison
65,469 PointsIf you'd like to learn about Python list comprehensions, you could check out this workshop.
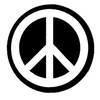
john larson
16,594 PointsHi Fahad, I looked at your code. Very nice. Was it a bit of a process to get to that? It took me a minute to see that you called one function from inside the other.

fahad lashari
7,693 PointsHi john. Yes it did take me a while to get my head around the task as I had the same problem as many other people: the programme wouldn't take out every single vowel from every single word so the strategy that worked best for me was to separate out the loops into two different functions and call the other function inside a loop. This way I wouldn't actually be taking the vowels out but I would be creating new words without the vowels and the loop for each run would run until it iterated through all the letters in the word, solving the problem of missing vowels. Kind of the opposite solution to Kenneth's one but it seemed to have worked lol
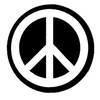
john larson
16,594 PointsHey Fahad, I got an idea looking at yours. I tried to do a similar thing, only REMOVING the vowels. But it's only doing the first word. Can you see why? Thanks for any help.
words = ["first", "second", "third", "fourth", "fifth"]
def vowelless(words):
words_without_vowels = []
for word in words:
word_as_list = list(word)
for letter in word_as_list:
if letter in "aeiou":
word_as_list.remove(letter)
new_word = " ".join(word_as_list)
words_without_vowels.append(new_word)
return words_without_vowels
print(vowelless(words))

fahad lashari
7,693 PointsHi john. I believe the 'return' statement being inside the loop is ending the loop after only one word. Just unindent the return statement before the first loop and that should fix the early ending problem. The return statement is kind of like a break statement for functions. As soon as a programme encounters a return statement, it stops all loops and return the object. And as for the vowel removal, the loop is still missing some vowels, I had this exact same problem, hence why I decided to take the opposite approach of creating new words without vowels. If you figure out the removal solution please post your code and share it.
Even better, you try out a few things and post your code and I'll try to add a few things and we'll go back and fourth until we solve this! :D

fahad lashari
7,693 PointsHi john. I actually had a good crack at the problem and figured out a REMOVAL method. Using a while loop with a try and except did the trick as it ensured the loop kept running until all vowels were removed and then broke the while loop in the except once there were no more vowels left and moved onto to the new word.
Here is the solution:
words = ["first", "second", "third", "fourth", "fifth"]
vowels = list('aeiou')
def vowelless(words):
new_word = ""
words_without_vowels = []
for word in words:
word_as_list = list(word)
for letter in vowels:
while True:
try:
word_as_list.remove(letter)
except:
break
new_word = " ".join(word_as_list)
words_without_vowels.append(new_word)
print(words_without_vowels)
vowelless(words)
Hope this helps

fahad lashari
7,693 PointsHi Alex. That is a very neat way of achieving the task. Impressive. I will most certainly have a look at that tutorial and get up to date with list comprehension. I can second John on the list comprehension not being even mention in the course so far. Thanks for providing the link
john larson
16,594 Pointsjohn larson
16,594 PointsYes, your exactly right. moving the return fixed the problem of only one word being in the list. And revealed that not all the vowels were removed. Time for bed soon. Thanks. I'll take another look tomorrow.