Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial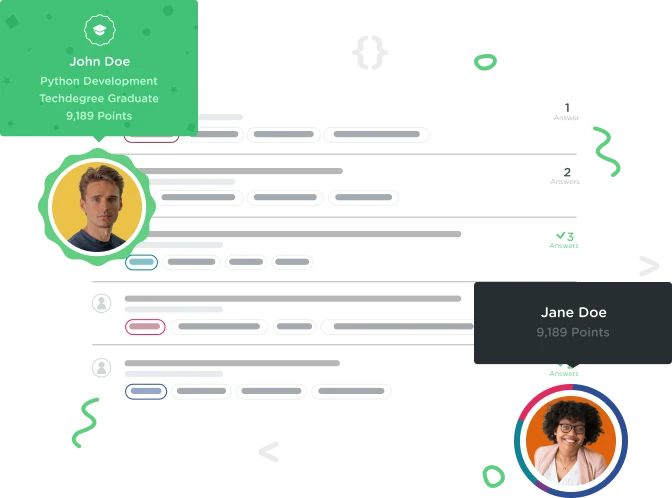
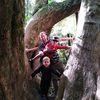
Brett McGregor
Courses Plus Student 1,903 PointsHere is my solution to opposite number game. I used a sort of binary search rather than generating random numbers.
import random
import math
def game():
#set number range
lower = 1
upper = 100
# track number of attempts
attempts = 0
#Introduction message describing the game
print("Hi. I am your computer.\nThink of a number between {} and {}.\n\
I will make a guess.\nThen please tell me if my guess is high or low.\
\nOkay, I will make a guess:".format(lower, upper))
while True:
#Computer guesses a number, it is printed
guess = math.floor(((upper + lower) / 2))
attempts += 1
print(guess)
# User input, telling computer if its guess is high or low or correct
user_feedback = str.upper(input("High(h)/Low(l)/Correct(c)? > "))
if user_feedback == "H":
upper = guess
elif user_feedback == "L":
lower = guess
elif user_feedback == "C":
print("Woohoo!!! I guessed your number \
which was {}. It took me {} attempts.".format(guess, attempts))
# play again?
replay = str.upper(input("Would you like to play again? Y/n > "))
if replay == "N":
print("OK. See you next time.")
break
else:
game()
# handle incorrect user feedback entries
else:
if user_feedback != "H" or "L" or "C":
print("please enter either H, L or C")
attempts -= 1
game()
1 Answer
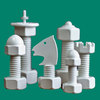
Steven Parker
231,275 PointsGood idea — I did the same thing when I took that course! This way, the computer always gets the number with the minimum number of guesses.