Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial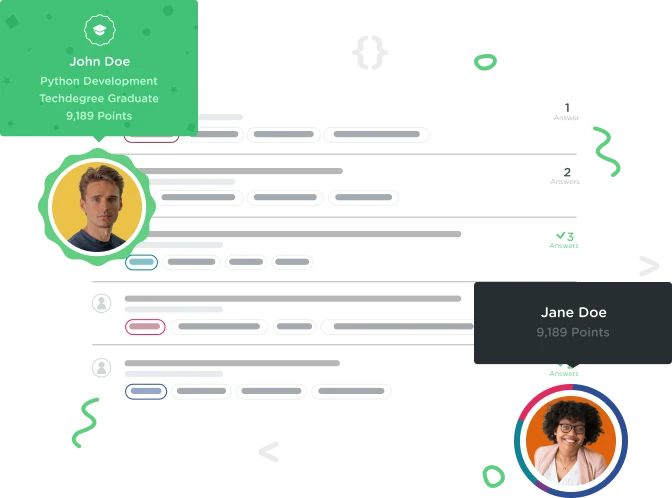

MANUEL CERNA
Courses Plus Student 4,739 PointsHere is my solution to this challenge. A took the liberty of having some fun with this challenge.
js script below: ------------------- BEGIN --------------
//Declare variables that will be used in loops var html = ''; var red; var green; var blue; var rgbColor; var counter = 0;
//Create two loops. A for loop is created within a while loop.
//This provides for some interesting sets of divs.
//While loop iterating 2 times to create two sets of 10 divs.
//The 10 divs are created using the for loop. See * below.
//I edited the CSS file to include text within each div that spells: "M&M"
//They sort of look like M&M's!
while (counter < 2) {
counter += 1;
document.write("<h1> ----Increment while loop 2 times (Begin)------- </h1>")
// * For loop iterating 10 times to create 10 different colored divs using the
//Math.random number generator method
for (var i = 1; i <= 10; i+= 1) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"><h2>M&M</h2></div>';
}
//Creat output of results using document.write function that uses html as its parameter document.write(html); document.write("<h1> ----Increment for loop 10 times (End)--------------- </h1>")
--------------------END--------------------
css file (only included the new div that created the "M&M", text within div
color h2 {
text-align:center; line-height: 25px; vertical-align: middle; }
4 Answers
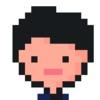
Owa Aquino
19,277 PointsGreat work Manuel, Here's my solution.
var html = '';
var red;
var green;
var blue;
var rgbColor;
for (var i = 1; i<10; i+=1){
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
console.log('Color value : ' + rgbColor);
}
document.write(html);
Cheers!

Martin Krkoska
10,514 PointsHi I am new here, I hope it is ok to put here my solution too ;-)
var html = '';
/* random number 0-255 */
function randColorNum () {
return Math.floor(Math.random() * 256 );
}
/* rgb = random red , random green , random blue */
function randRGB () {
return 'rgb(' + randColorNum () + ',' + randColorNum () + ',' + randColorNum () + ')';
}
for ( var i = 0; i < 10; i += 1) {
html += '<div style="background-color:' + randRGB () + '"></div>';
}
document.write(html);

MANUEL CERNA
Courses Plus Student 4,739 PointsYes, that is great. Thanks.

Adam Lopez
3,475 Pointsfor (i=0, i>10, i++) {
document.write('<div style="background-color:' + 'rgb(' + Math.floor(Math.random() * 256 ) + ',' + Math.floor(Math.random() * 256 ) + ',' + Math.floor(Math.random() * 256 ) + ')' + '"></div>');
}
Pretty sure this is the shortest way of doing it.

MANUEL CERNA
Courses Plus Student 4,739 PointsAdam, thanks for the post. I will add this code and test out. Thanks.
MANUEL CERNA
Courses Plus Student 4,739 PointsMANUEL CERNA
Courses Plus Student 4,739 PointsGreat work Owa!