Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial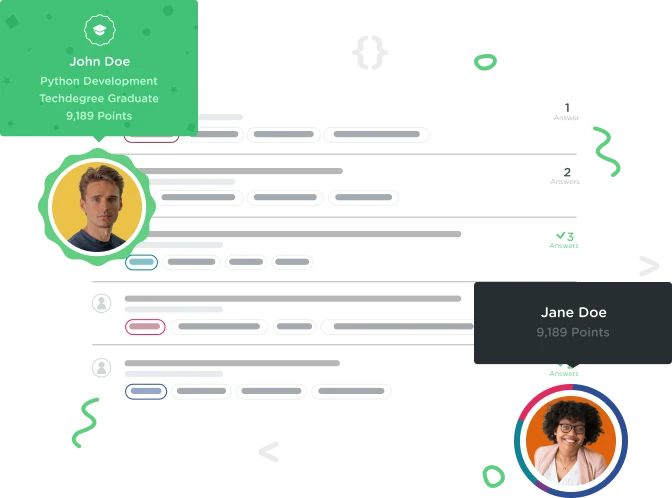

fahad lashari
7,693 PointsHere is the 'del' function implemented into shopping_list_3. Do let me know if this helps you. thanks
import sys
shopping_list = []
multiple_items = []
print("""Would you like to start your supreme AI shopping experience?\n Y/n""")
command = input('>')
def show_help():
print('\nSeperate each item with a comma.')
print('\nType DONE to quit, SHOW to see the current list, DELETE to see the delete menu and HELP to get this message')
add_item()
def show_list():
count = 1
for item in shopping_list:
print('{}: {}'.format(count, item))
count+=1
print("Continue adding items? Y/n")
continue_adding = input('>')
if continue_adding == "Y".lower():
add_item()
else:
print("Good bye!")
def delete_item():
print("Please type the index number of the item you would like to delete")
print("For example to delete '{}' type {}".format(shopping_list[0], '1'))
count = 1
for item in shopping_list:
print('{}: {}'.format(count, item))
count+=1
delete = input('>')
del shopping_list[int(delete)-1]
add_item()
def add_item():
print('\nPlease enter the name of the item or enter a COMMAND\nType HELP to see the available commands\nIf more than one item, Seperate each item with a comma: \n')
command = input('>')
if command != "DONE".lower() and command != "SHOW".lower() and command != "HELP".lower() and command != "DELETE".lower():
print("Add this to certain spot on the list?, "
"\ncurrently we are on number {}\nPRESS ENTER for last spot and continue or give me a NUMBER. NO LETTERS or " "SYMBOLS:\n".format(len(shopping_list)+1))
index = input('>')
new_list = command.split(",")
if index:
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot+=1
else:
for item in new_list:
shopping_list.append(item.strip())
add_item()
else:
function_dict[command]()
def exit():
print("""Thank for shopping with us.
I know it's a bit late but we dont really sell anything...\nThe shopping list looks neat though!\nOk bye now""")
sys.exit()
function_dict = {"Y".lower():add_item, "HELP".lower():show_help, "SHOW".lower():show_list, "DELETE".lower():delete_item, "DONE".lower():exit}
function_dict[command]()