Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial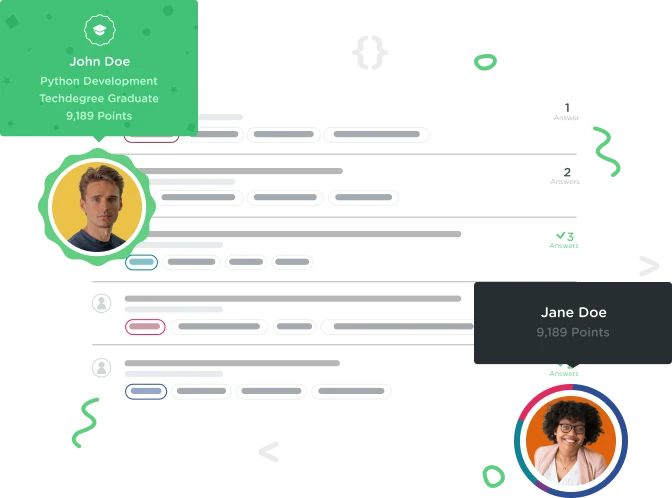

Amy Shah
25,337 PointsHere is what I attempted I am getting an error that says I did not call the OnMap method
I though I did try to call the OnMap method but it is not working
namespace TreehouseDefense
{
public class Map
{
public readonly int Width;
public readonly int Height;
public Map(int width, int height)
{
if(width < 1 || height < 1)
{
throw new System.ArgumentOutOfRangeException(
"Map must be at least 1x1");
}
Width = width;
Height = height;
}
public bool OnMap(Point point)
{
return point.X >= 0 && point.X < Width &&
point.Y >= 0 && point.Y < Height;
}
}
}
using Xunit;
namespace TreehouseDefense.Tests
{
public class MapTests
{
[Fact]
public void OnMapTest()
{
Assert.True(false, "This test needs an implementation");
}
[Fact]
public void OnMap()
{
Assert.True(true, "sdfaf");
}
}
}
using System;
namespace TreehouseDefense
{
public class Point
{
public readonly int X;
public readonly int Y;
public Point(int x, int y)
{
X = x;
Y = y;
}
public double DistanceTo(Point point)
{
return Math.Sqrt(Math.Pow(X - point.X, 2.0) + Math.Pow(Y - point.Y, 2.0));
}
}
}
1 Answer

Mohammad Laif
Courses Plus Student 22,297 PointsYou need to create a point and a map. Then call the onMap() from your map object and pass your point there. FYI, your point need to be inside your map range, so it could return true.
using Xunit;
namespace TreehouseDefense.Tests
{
public class MapTests
{
[Fact]
public void OnMapTest()
{
var myPoint = new Point(1,1);
var myMap = new Map(4,4);
Assert.True(myMap.OnMap(myPoint), "This test needs an implementation");
}
}
}