Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial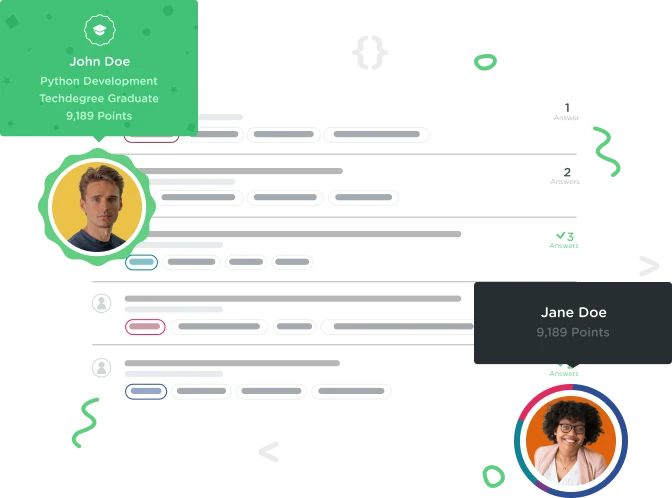
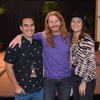
Ty Yamaguchi
25,397 PointsHere's how I did it :)
function print(message) {
document.write(message);
}
var numCorrect = 0;
var resultsHTML = '';
var correctHTML = '<h2>You got these questions correct:</h2><ol>';
var incorrectHTML = '<h2>You got these questions wrong:</h2><ol>';
var questions = [
[ 'How many states are in the United States?', 50 ],
[ 'How many legs does an insect have?', 6 ],
[ 'How many continent are there?', 7 ]
];
//ask questions by looping through questions
for ( var i = 0; i < questions.length; i++) {
//get the question from array
var question = questions[i][0];
//get the answer from array
var correctAnswer = questions[i][1];
//prompt user for answer to question
var userAnswer = prompt(question);
userAnswer = parseInt(userAnswer);
//evaluate userAnswer
if ( userAnswer === correctAnswer ) {
// add 1 to numCorrect
numCorrect++;
// add question to correctHTML as a list item
correctHTML += '<li>' + question + '</li>';
} else {
// add question to incorrectHTML as a list item
incorrectHTML += '<li>' + question + '</li>';
}
}
correctHTML += '</ol>';
incorrectHTML += '</ol>';
if ( numCorrect && numCorrect === questions.length ) {
resultsHTML += '<p>You got all ' + questions.length + ' questions right!</p>';
} else if ( numCorrect ) {
resultsHTML += '<p>You got ' + numCorrect + ' question(s) right.</p>';
} else {
resultsHTML += '<p>You got them all wrong!</p>';
}
if ( numCorrect ) {
resultsHTML += correctHTML;
}
if ( numCorrect < questions.length ) {
resultsHTML += incorrectHTML;
}
print(resultsHTML);