Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial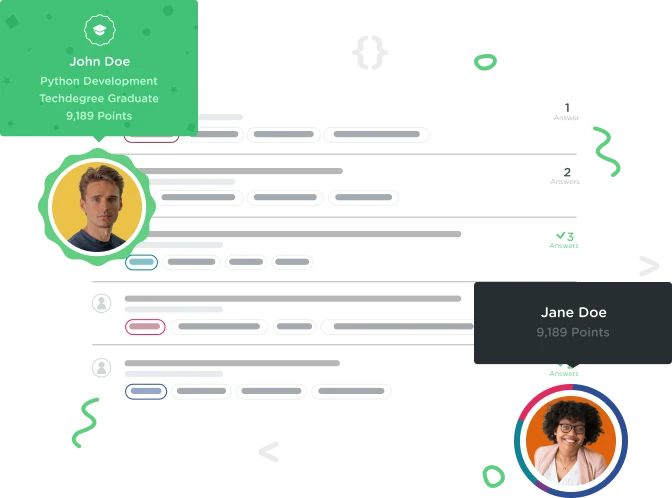

Yuval Shechter
11,716 PointsHere's my 25 lines solution for those who may be interested:
var correctAnswers = 0;
var wrongAnswers = 0;
function print(message) {
document.write(message);
}
var questions = [
["What is the capital of New Zealand?", "auckland"],
["What is the capital of Israel?", "jerusalem"],
["What is the capital of England?", "london"]
];
for (var i = 0; i < questions.length; i++) {
var question = prompt(questions[i][0]);
if (question.toLowerCase() == questions[i][1]) {
correctAnswers++;
} else {
wrongAnswers++;
}
}
var message = "<p> You've got " + correctAnswers + " correct answers and " + wrongAnswers + " wrong answers. </p>";
print(message);
3 Answers

Markus Ylisiurunen
15,034 PointsLooks great! Good job.

Tom Goldsmith
1,410 PointsI agree with Markus, it's a good solution to the problem in the course, answered in the way expected. The print function was actually provided as part of the workspace, so pretty understandable/ it was expected to be used XSS issues or not.

Jacob Mishkin
23,118 PointsThe code looks great, but lets level up!
first, rewrite the print function using Node.textContent you can find out more here textContent in the courses ahead they will teach you something called innerHTML to print to the DOM, and due to possible XSS attacks, I would advise you to use textContent when printing to the DOM.
Second, so you have this variable message, why not make that variable a div, in your HTML, and then style that div so when you take the quiz it looks a lot nicer then just plain text.
As of now the code looks good, but why not level up, the more you know the more you can do.
if you want to know more about an XSS attack please read here XSS

Markus Ylisiurunen
15,034 PointsYou're right about what you said but I honestly think it's way too advanced at this point to talk about cross-site scripting attacks. They are not something you should be worrying when starting to learn coding. You'd need to know a lot more about how the web works and how XSS attacks work to understand why it's better to use textContent or innerHTML.
As I said you're correct about what you said but it's not relevant at this point and it probably just would take the focus off from learning basic coding.

Jacob Mishkin
23,118 PointsMarkus Y, I understand were you are coming from, but I have to disagree with you to some level. I think we both agree in that understanding cross-site scripting attacks is more on the advanced side of things, I agree, It may not be necessary to be taught, but just because it's advanced, doesn't mean that someone should not be aware of its existence. The motivation behind using textContent is so he can level up, from using the doc.write method, it was not meant to be a derailment from learning basic code, but rather letting someone know of a more advanced but still attainable way to do the same function.
It is relevant, in that if and I stress if, the code using innerHTML or something of its likeness goes live, there is a possibility of issues, the likeliness of something happening, rare, but I do not feel that there is an issue, letting someone know of potential issues, and also introducing something new, that is obtainable, and not out of context. Yuval's code is sweet, but there's nothing wrong with leveling up.