Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial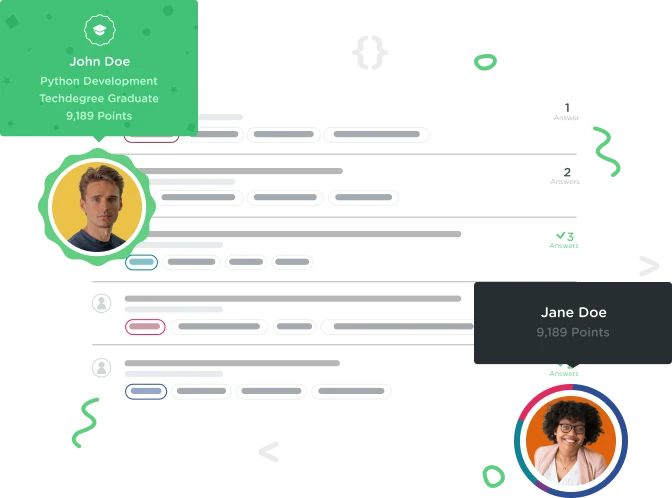
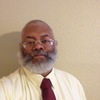
Adiv Abramson
6,919 PointsHere's my attempt at the challenge. OK, it's probably overkill but I had fun doing it. :-)
In my solution, each element of the quizdata array is itself an array consisting of the question, the answer, whether the question has been answered correctly and the number of attempts to answer the question.
The program prompts the user for an answer up to three times for a given question. When all three questions have been attempted a congratulatory message is displayed if all questions were answered correctly. A summary of the number of attempts at each question and whether it was answered correctly is printed to the web page.
//create 2D array [question, answer, guessedCorrectly, #times guessed]
var quizdata = [];
//populate quizdata and prompt user to play or quit
setupQuiz();
function print(message) {
document.writeln(message + "<br />");
}
function msgAsk(strMessage) {
return confirm(strMessage);
};//msgAsk
function displayStats() {
var strStats = "";
for (var i = 0; i < quizdata.length; i++) {
strStats = "You guessed question #" + (i + 1)
+ " " + ((quizdata[i][2]) ? "correctly" : "incorrectly")
+ " after " + parseInt(quizdata[i][3]) + " attempts.";
print(strStats);
}//(var i = 0; i < quizdata.length; i++)
};//displayStats()
function getCorrectAnswerCount() {
/*increment count of correctly answered questions when
element 2 of associated array is true */
var count = 0;
for (var i = 0; i < quizdata.length; i++) {
if (quizdata[i][2]) {
count++;
}//if (quizdata[i][2])
}//for
return count;
};//getCorrectAnswerCount()
function setupQuiz() {
//clear array of any data
quizdata.length = 0;
//insert an array consisting of a question and then answer, guessedCorrectly and timesGuessed
//guessedCorrectly default is false; timesGuessed default is 0
var strQuestion = "";
strQuestion = "How many planets are there in the solar system?";
strAnswer = "8";
quizdata.push([strQuestion, strAnswer, false, 0]);
strQuestion = "What is the most prevalent element in the earth's atmosphere?";
strAnswer = "Nitrogen";
quizdata.push([strQuestion, strAnswer, false, 0]);
strQuestion = "Is Python strongly typed?";
strAnswer = "No";
quizdata.push([strQuestion, strAnswer, false, 0]);
//welcome the user to the quiz
print("Welcome to the Quiz Game!");
print("You have 10 tries to answer three questions.");
print("You can type STOP! at any prompt to end the game immediately.");
if (!msgAsk("Ready to play?")) {
alert("Thank you!");
return;
} else {
runQuiz();
}
};//setupQuiz()
function askQuestion() {
//display first incorrectly or unaswered question available.
//don't display correctly answered question
//check user input aganst element 1 of array
//return true if answered correctly; otherwise return false
var currentQuestion = 0; //index of next incorrectly answered question
//get index of next incorrectly answered question attempted no more than three times
for (var i = 0; i < quizdata.length; i++) {
if (!quizdata[i][2] && quizdata[i][3] < 3) {
currentQuestion = i;
break;
}//if (!quizdata[i][2])
}//(var i = 0; i < quizdata.length; i++)
while (quizdata[currentQuestion][3] < 3) {
//increment count of attempts to answer this question
quizdata[currentQuestion][3]++;
if (prompt(quizdata[currentQuestion][0]).toLowerCase() === quizdata[currentQuestion][1].toLowerCase()) {
//mark question as correctly answered
quizdata[currentQuestion][2] = true;
alert("Great! You answered the question correctly!");
break;
} else {
alert("Oops! That is not the correct answer. " + (3 - quizdata[currentQuestion][3]) + " tries remaining");
//ask again until timesGuessed >= 3
}//if (prompt(quizdata[currentQuestion][0]) === quizdata[currentQuestion][1].toLowerCase())
}//while (quizdata[currentQuestion][3] <= 3)
return;
};//askQuestion()
function runQuiz () {
/*run while loop until one of the following is true:
1) all three questions have been answered coreectly
2) user inputs "STOP!"
3) maxTries is exceeded */
var maxTries = 10;
var tryCount = 0;
while (true) {
if (tryCount < maxTries) {
tryCount++;
} else {
alert("GAME OVER!");
break;
}//if (tryCount < maxTries)
//check number of correctly answered questions
if (getCorrectAnswerCount() === 3) {
alert("You won the game by answering all three questions correctly!\nThank you for playing!");
break;
}//if (getCorrectAnswerCount() === 3)
askQuestion()
}//while
//let user know how well he/she did
displayStats();
return;
};//runQuiz()
1 Answer
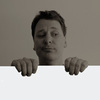
Sean T. Unwin
28,690 PointsGreat job with the commenting. I particularly like the closing bracket comments; they help a lot. I use those, too, especially closing div
s in HTML.
I would suggest declaring the strAnswer
variable inside the setQuiz()
function. By not explicitly declaring a local variable inside a function, that variable is placed in the global scope. You have declared strQuestion
so that's good, just declare the other one below the first.
Try to keep your indenting consistant as well.
Super effort. :)
Adiv Abramson
6,919 PointsAdiv Abramson
6,919 PointsThank you so much! I hadn't noticed that I failed to formally declare the strAnswer variable until just now, as I read your comment. Thank you for pointing out this error! I am used to working with VB(A|6|.NET) with the Option Explicit setting that requires all variables to be declared, otherwise the script will not compile. Do you know if JS has a similar setting?
Sorry about any inconsistent indenting. Do you know if WorksSpace or any other JS editor can apply proper indentation? Again, in the VBx editors I'm used to the indentation is done automatically.
Thanks again!
Sean T. Unwin
28,690 PointsSean T. Unwin
28,690 PointsYou can use JSLint online or install JSHint to run locally on your computer via Nodejs. Furthermore, many development-style text editors have some form of a JSHint plugin. Each of these will format your code as well as attempt to catch any errors. There are also quite a few configuration options to choose from, although very simple to use.