Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial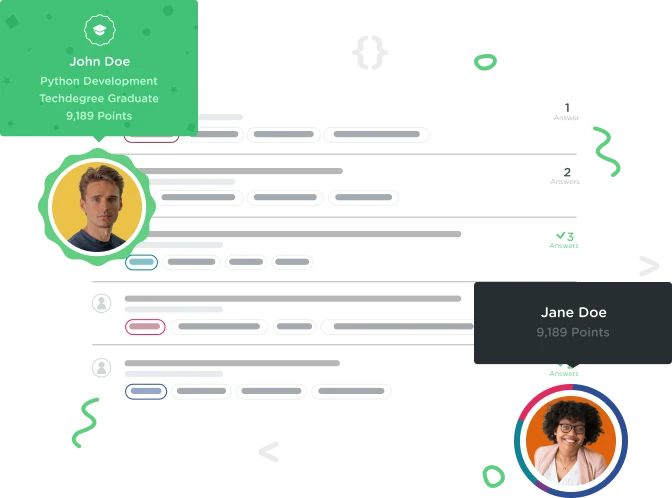

Dzmitry Aliakseichyk
12,290 PointsHere's my attempt at the Solution ¯\_(ツ)_/¯
Ok this one was the hardest step BY FAR. It took me not too much time to come up with the first raw solution that worked. But then it took a lot longer and 2 more versions to polish everything and comply with the DRY. Gotta mention that in the step playToken()
I went a little sideways and made that method to assign the space being filled with the Player's ID, so each taken space holds that value (1 or 2) inside owner property instead of null. Then checkForWin()
receives a parameter which is token being played. I skipped get owner()
and mark()
because there's no need of them in this version.
playToken() {
let spaces = this.board.spaces;
let activeToken = this.activePlayer.activeToken;
for (let r = this.board.rows - 1, c = activeToken.columnLocation; r >= 0; r--) {
if (spaces[c][r].token === null) {
// this.ready = false;
spaces[c][r].token = activeToken.owner.id;
activeToken.drop(spaces[c][r]);
this.checkForWin(spaces[c][r]);
break;
}
}
}
checkForWin(spaceId) {
let spaceID = spaceId.token;
let col = spaceId.x;
let row = spaceId.y;
let spaces = this.board.spaces;
//HORIZONTAL
for (let c = col - 3; c <= col; c++) {
for (let n = 0; n <= 3; n++) {
if (typeof spaces[c + n] === 'undefined' ||
spaces[c + n][row].token !== spaceID) {
break;
} else if (n === 3) {
console.log('CONGRATS');
}
}
}
//VERTICAL
for (let r = row - 3; r <= row; r++) {
for (let n = 0; n <= 3; n++) {
if (typeof spaces[col][r + n] === 'undefined' ||
spaces[col][r + n].token !== spaceID) {
break;
} else if (n === 3) {
console.log('CONGRATS');
}
}
}
//DIAGONAL 'BACKSLASH'
for (let r = row - 3, c = col - 3; r <= row, c <= col; r++, c++) {
for (let n = 0; n <= 3; n++) {
if (typeof spaces[c + n] === 'undefined' ||
typeof spaces[col][r + n] === 'undefined' ||
spaces[c + n][r + n].token !== spaceID) {
break;
} else if (n === 3) {
console.log('CONGRATS');
}
}
}
//DIAGONAL 'SLASH'
for (let r = row + 3, c = col - 3; r >= 0, c <= col; r--, c++) {
for (let n = 0; n <= 3; n++) {
if (typeof spaces[c + n] === 'undefined' ||
typeof spaces[col][r - n] === 'undefined' ||
spaces[c + n][r - n].token !== spaceID) {
break;
} else if (n === 3) {
console.log('CONGRATS');
}
}
}
}
Steven Parker
231,275 PointsSteven Parker
231,275 PointsIf you want to make it possible for folks to try out your project, you can make a snapshot of your workspace and post the link to it here.