Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial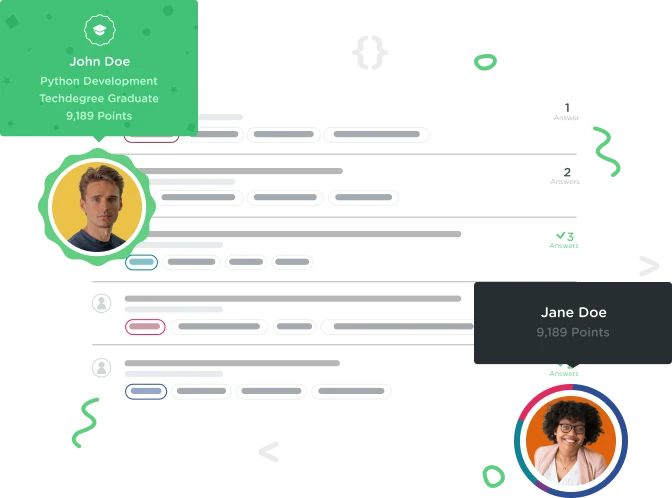
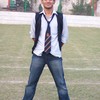
Shivang Chaturvedi
2,600 PointsHere's my code
var fName = prompt("Enter your first name"); var str_fname = fName.length; var lName = prompt("Enter your last name"); var str_lname = lName.length; var space = " ";
var name = fName.toUpperCase()+ space + lName.toUpperCase(); var str = str_fname + str_lname; console.log(str);
var message = "The string " + name;
message += " is " + str + " characters long."; alert(message);
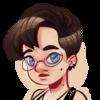
Alexandra Wakefield
1,866 PointsWhoa, Cosimo, I love how you organized your solution! I had no idea how to insert more than one action in a function; actually, it didn't even cross my mind that we could.
Here's my solution:
var firstName = prompt("What is your first name?");
var lastName = prompt("What is your last name?");
var fullName = firstName.toUpperCase() + " " + lastName.toUpperCase();
console.log(fullName);
var nameLength = fullName.length;
console.log(nameLength);
alert("The string " + fullName + " is " + nameLength + " characters long.")
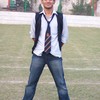
Shivang Chaturvedi
2,600 PointsThank you so much for your brilliant answers and suggestions.
11 Answers
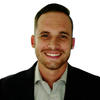
Joshua Petry
6,313 PointsHi Guys! Here's my code:
let firstName = prompt("What is your first name?");
let lastName = prompt("What is your last name?");
let fullName = (firstName + " " + lastName).toUpperCase();
let nameCharLength = fullName.length;
alert("The string '" + fullName + "' is " + nameCharLength + " characters long.");
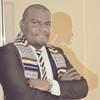
Houssein Abil
Full Stack JavaScript Techdegree Student 1,047 PointsHi, your answers are good but you miss to add the doudble quote around the name like in the video
var firstName = prompt("What is your first name");
var lastName = prompt("What is your last name");
var fullName = (firstName +" "+ lastName);
var fullName = fullName.toUpperCase();
console.log(fullName.toUpperCase());
console.log(fullName.length);
var fullNameNumber = fullName.length;
alert("Your name \"" + fullName + "\" contains " + fullNameNumber + " Characters");

abdirahim Maslah
2,060 PointsThis is how i did it, and i have added few extras at the end.
var firstName = prompt("What is your first name ?"); var lastName = prompt("What is your last name ? "); var fullName = firstName + lastName ; var nameLength = fullName.length; alert ("the name " + fullName + " is " + nameLength + " characters long " ); document.write("the name " + fullName + " is " + nameLength + " characters long " ); document.write("Mohamed is geneius, he is loving javascript ");
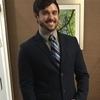
andressardou
Full Stack JavaScript Techdegree Student 3,218 Pointsvar firstName = prompt("What is your first name?");
var lastName = prompt("What is your last name?");
var fullName = firstName.toUpperCase() + ' ' + lastName.toUpperCase();
var nameLength = fullName.length;
var message = "Your name " + fullName;
message += " is " + nameLength;
message += " characters long.";
alert(message);

Timothy Shores
4,501 PointsI'm not sure if I am missing anything but I thought that I would share my solution as well.
var input1 = prompt("Enter an input");
var input2 = prompt("Enter another input");
uppercase = input1.toUpperCase() + " " + input2.toUpperCase();
alert("The string " input1.toUpperCase() + " " + input2.toUpperCase() " is " + uppercase.length + " number of characters long.");
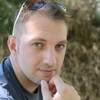
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 Pointsvar firstName = prompt("What is your First Name?");
var lastName = prompt("What is your Last Name?");
var fullName = firstName.toUpperCase();
fullName += " " + lastName.toUpperCase();
fullName += " SALLY FORTH";
var num1 =fullName.length;
document.write(fullName);
alert("the string " + fullName + " is " + num1 + " characters long");
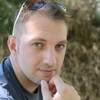
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 Pointsgot the results I needed, any suggestions to optimise my code?
document.write was not required for this just added it for testing purposes on my side.

ingridg
4,728 PointsHere is my code
var favFood = prompt("What is your favorite food?"); var favSong = prompt("Name your favorite song"); var superHero = "Your super hero name is: " var message = favFood + " " + favSong; alert(superHero + message.toUpperCase()); var lengthNum = message alert("The length of your super hero name, " + message + " is: " + lengthNum.length);
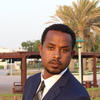
Yonas Fesehatsion
Full Stack JavaScript Techdegree Student 7,950 Pointsvar firstName = prompt('What is your first name?');
var lastName = prompt('What is your last name?');
var fullName = firstName.toUpperCase() + ' ' + lastName.toUpperCase()
var numberFullname = fullName.length
alert('The string ' + fullName + ' is ' + numberFullname + ' characters long.');
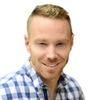
professionalb
Full Stack JavaScript Techdegree Student 5,082 Points
Jessica Hoang
1,243 PointsHere's My code:
// First and second question for the user:
const question1 = prompt("What is your favorite animated show?");
const question2 = prompt("What is the name of your favorite character from that show?");
// Combine the answers into one string. Include a space inbetween
const combinedAnswer = `${question1.toUpperCase()} ${question2.toUpperCase()}`;
// Find the length of combinedAnswer and store it in a variable
const lengthOfAnswer = combinedAnswer.length;
// Type it into the statement: 'The string "___" is __ characters long.'
// Than display it as an alert pop up
const yourInput = `The string "${combinedAnswer}" is ${lengthOfAnswer} characters long. `;
const finalAlert = alert(yourInput);

scott knudsen
768 PointsHere is my code, var firstName = prompt('What is your first name? '); var lastName = prompt(' What is your last name?'); var upperCase = firstName.toUpperCase() + " " + lastName.toUpperCase(); var strLength = upperCase.length; var sentence = "The string " + upperCase + " is " + strLength + " number of characters long."; document.write(sentence);
Cosimo Scarpa
14,047 PointsCosimo Scarpa
14,047 PointsNice but about this part:
var str_lname = lName.length; var space = " ";
It's better put the variables in two separate lines for less mess.
Anyway this is my solution