Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial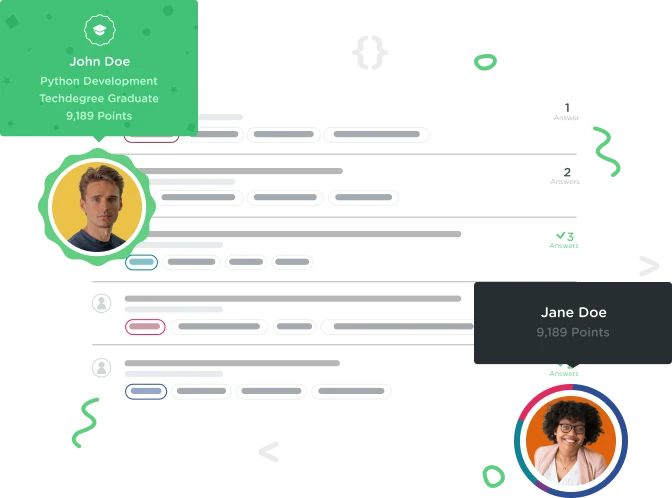
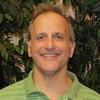
Robert Glover
20,115 PointsHere's my error: We tried your spec with a version of clone.js that DOESN'T work correctly, expecting the test to fail.
Here's my error: We tried your spec with a version of clone.js that DOESN'T work correctly, expecting the test to fail.
Here's my code:
function clone (objectForCloning) { return Object.assign({}, objectForCloning); }
module.exports.clone = clone;
var expect = require('chai').expect;
describe('clone', function () { var clone = require('./clone.js').clone; it('Returns property match', function () {
// YOUR CODE HERE
let obj1 = {
val1: 1,
val2: 2
};
let obj2 = clone(obj1);
//expect({val1:1, val2:2}).to.deep.equal(obj1);
expect(obj2).to.deep.equal(obj1);
});
});
What's the problem?
var expect = require('chai').expect;
describe('clone', function () {
var clone = require('./clone.js').clone;
it('Returns property match', function () {
// YOUR CODE HERE
let obj1 = {
val1: 1,
val2: 2
};
let obj2 = clone(obj1);
//expect({val1:1, val2:2}).to.deep.equal(obj1);
expect(obj2).to.deep.equal(obj1);
});
});
function clone (objectForCloning) {
return Object.assign({}, objectForCloning);
}
module.exports.clone = clone;
7 Answers
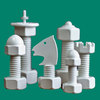
Steven Parker
231,275 PointsTwo suggestions:
- don't modify the code in "clone.js"
- change your declarations to use "var" or "const" instead of "let"
I have no idea why that last one makes a difference, but it does!
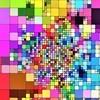
james south
Front End Web Development Techdegree Graduate 33,271 Pointsthey are not asking for object equality here, just that the properties are the same. so you don't need equal.
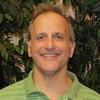
Robert Glover
20,115 PointsI changed to:
expect(obj2).to.have.property('val1');
expect(obj2).to.have.property('val2');
Same error. (I have a separate test environment, so I know that these pass on my laptop environment.) I'm not sure wgat they're asking for.
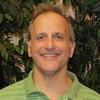
Robert Glover
20,115 PointsCurrent code:
var expect = require('chai').expect
describe('clone', function () { var clone = require('./clone.js') it('Returns object property match', function () {
// YOUR CODE HERE
var obj1 = {
val1: 1,
val2: 2
};
var obj2 = clone(obj1);
// Add expectation
expect(obj2).to.have.property('val1');
expect(obj2).to.have.property('val2');
})
And in clone.js (no changes):
function clone (objectForCloning) { return Object.assign({}, objectForCloning) }
module.exports = clone
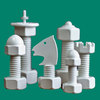
Steven Parker
231,275 PointsTry your original code with my suggestions applied (it passed for me).
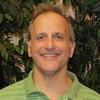
Robert Glover
20,115 PointsOkay, I'm going to shoot myself. That code now works. Thanks, Steven and James!
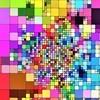
james south
Front End Web Development Techdegree Graduate 33,271 Pointswow it must accept several answers, I passed using the keys method.
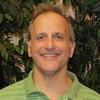
Robert Glover
20,115 PointsFinley, long time ago. I had to refresh my memory. Treehouse is picky. You have to use var instead of let or const.
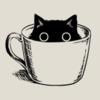
Finley Williamson
11,375 PointsYeah I was only worried that there was some programmatic difference that I wasn't aware of. Thanks for the response
Finley Williamson
11,375 PointsFinley Williamson
11,375 PointsI would love to know why this worked... after trying several different method combinations and getting this ^^ error, changing my 'let' to 'const' cleared the challenge.