Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial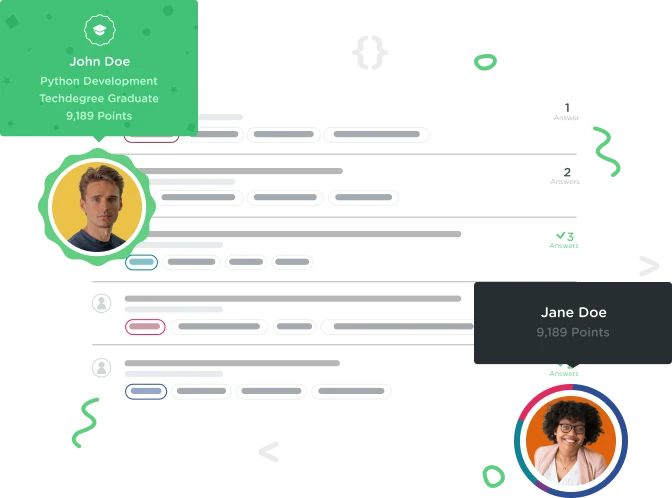

Adrien Contee
4,875 PointsHere's my solution to part 1 of the extra credit. (Printing a student not found message to the screen)
FYI I always declare my functions first and then my variables. Even if the function is using a variable that isn't declared until later on in the code it still works properly because of hoisting and because of how the browsers' javascript interpreter parses a javascript document.
All this you already know, but I'll add anyway for reference:
function print(message){
var div = document.getElementById("write");
div.innerHTML = message;
}
function studentRecords(student){
html = "<h2> Student: " + student.name + "</h2>";
html += "<p> Track: " + student.track + "</p>";
html += "<p> Achievements: " + student.achievements + "</p>";
html += "<p> Points: " + student.points + "</p>";
return html;
}
var students = [
{
name: "Tom",
track: "iOS Development",
achievements: 43,
points: 73839
},
{
name: "Jerry",
track: "Web Design",
achievements: 23,
points: 89498
},
{
name: "Sam",
track: "Front End Development",
achievements: 86,
points: 23187
},
{
name: "Kim",
track: "Back End Development",
achievements: 24,
points: 90594
},
{
name: "Jane",
track: "Java Development",
achievements: 73,
points: 87328
}
];
var search;
var html = "";
Here's my solution:
// I first created an empty array to store the student names
var studentNames = [];
while(true){
search = prompt("Enter a Students Name: [or 'x' to quit]");
if (search === null || search.toLowerCase() === "x") {
break;
}
// I initially loop through the students array and push all the names to my studentNames array
for (var i = 0; i < students.length; i++) {
studentNames.push(students[i].name.toLowerCase());
}
// Now I use the name collected from the search prompt to check against the index number
// in the studentNames array using the .indexOf() method
if (studentNames.indexOf(search.toLowerCase()) > -1) {
// If the name exists in the studentNames array then send that as the argument for the studentRecords function
html += studentRecords(students[studentNames.indexOf(search)]); //studentNames.indexOf(search) returns a number. Which can be used as the index in students[#]
print(html);
} else {
// If the name isn't found then print this line to the screen
print("<h2>" + search + " not found</h2>");
}
// Because of the browsers quirk about leaving the prompt on the screen and not displaying
// the results until after you exit the prompt, I added a break after every search query
break;
}
Here's the code without comments. Might be easier on the eyes:
var studentNames = [];
while(true){
search = prompt("Enter a Students Name: [or 'x' to quit]");
if (search === null || search.toLowerCase() === "x") {
break;
}
for (var i = 0; i < students.length; i++) {
studentNames.push(students[i].name.toLowerCase());
}
if (studentNames.indexOf(search.toLowerCase()) > -1) {
html += studentRecords(students[studentNames.indexOf(search)]);
print(html);
} else {
print("<h2>" + search + " not found</h2>");
}
break;
}
It looks more complicated than it actually is. Hopefully I broke it down easy enough. No other way I tried worked for me, if someone else has a different solution I would love to see it.
Also I think I'm going to stop here and forgo tackling the second part of the extra credit which was to list all the students with the same name. I may change my mind however. But if someone comes up with a solution to that I would love to see it also.
Happy Coding.