Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial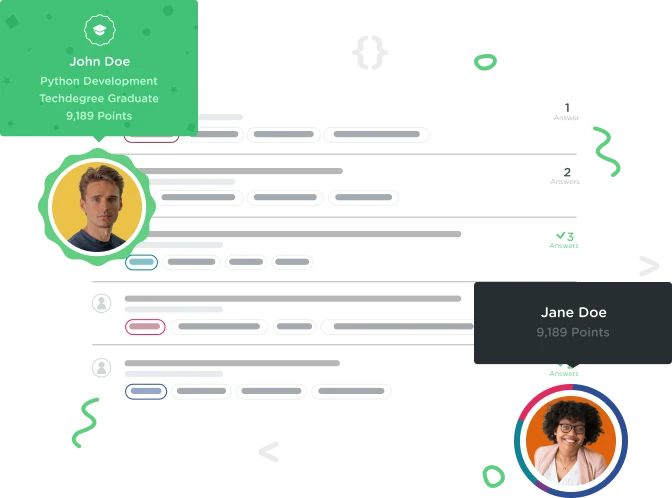
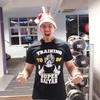
Jamie Cook
5,655 PointsHere's my solution to The Student Record Search Challenge. Additional challenges included and while loop not used.
Here's the snapshot of my solution https://w.trhou.se/ku7i2lkqya
I decided to try the challenge without the while loop. The solution will let you search for multiple students and display details of each one when the prompt is closed.
Comment if you see any errors or if you notice anything that could be improved. It's a bit different from what was shown in the video, so I thought it was worth posting.
2 Answers

Samuel Trejo
4,563 PointsHere is my solution, using what was taught up until now in this course. If name is not found it will print to document not found, and if there are duplicate names it will print both names and info. Hope someone finds this helpful was frustrating but fun to figure it out. Let me know if you have any feedback, or questions about it.
var students = [
{name: "Davy", track: "iOS", achievements: 5, points: 525},
{name: "Ito", track: "Web Design", achievements: 6, points: 25346},
{name: "Sami", track: "JavaScript", achievements: 7, points: 7467},
{name: "Dany", track: "Java", achievements: 9, points: 98769},
{name: "Davy", track: "iOS", achievements: 8, points: 13434}
];
var msg = "";
var student;
var search = "";
var name;
var notFound;
var counter = 0;
function print(msg){
var outputDiv = document.getElementById("output");
outputDiv.innerHTML = msg;
}
function getStudentData(student){
var report = "<h2>Student: " + student.name + "</h2>"
report += "<p>Track: " + student.track + "</p>"
report += "<p>achievements: " + student.achievements + "</p>"
report += "<p>points: " + student.points + "</p>"
return report
}
while(search.toLowerCase() !== "done"){
search = prompt("Type a name to search, to exit search type \"done\".");
for(i = 0; i < students.length; i++){
student = students[i];
counter++;
if(search.toLowerCase() === student.name.toLowerCase()){
msg = getStudentData(student);
notFound = false
print(msg);
break
}else if(search === "done"){
break
}else{
notFound = true
}
}
for(j = counter; j < students.length; j++){
student = students[j];
if(search.toLowerCase() === student.name.toLowerCase()){
msg += getStudentData(student);
print(msg);
}
}
if(notFound === true && msg === ""){
msg = "Sorry, no student found with that name."
print(msg)
search = "done"
}
print(msg)
}

Roger Hwang
3,851 PointsMy solution on 1st try factoring all the edge cases being searched for (didn't bother refactoring as it probably can be).
var element = document.getElementById("output");
while(true) {
var search = prompt('Enter student you want information for.');
if (search === null || search.toLowerCase() === 'quit') {
break;
}
else if (!students.find(item => item.Name.toLowerCase().includes(search.toLowerCase()))) {
alert('Could not find ' + search + ' in database.');
}
else if (search === "") {
alert('Please enter a student name.');
}
for (i = 0; i < students.length; i++) {
var database = students[i].Name.toLowerCase();
if (database.includes(search.toLowerCase()) && search !== "") {
for (var prop in students[i]) {
if(prop === 'Name') {
element.innerHTML = '<h2>' + prop + ': ' + students[i][prop] + '</h2>';
} else {element.insertAdjacentHTML('beforeend', '<div>' + prop + ': ' + students[i][prop] + '</div>')};
}
}
}
}