Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial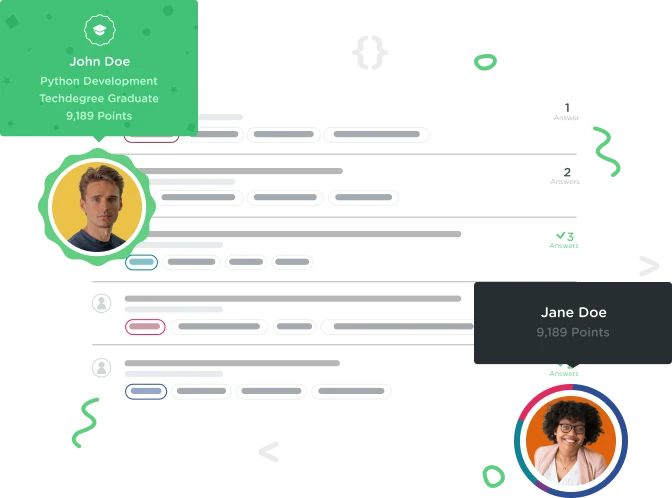

Jeremy Leeper
2,013 PointsHere's my try and fail! Ready to look now to see how this should be pulled off.
1 Answer
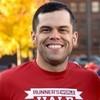
Juan Lopez
Treehouse Project ReviewerSo the challenge asked you to ask a user for 5 questions. Keep track of the ones they got right and at the end of the game depending on the number correct they get an assigned crown (gold, silver, bronze, or none). Using your code above We'll break it down in pieces.
var correctAnswers = 0; //assigns a starting value of 0 for correctAnswers
// Will store the answers to your "prompts" in questionOne thru questionFive
var questionOne = prompt("Hello, and lets begin the test! What is 2 x 2")
var questionTwo = prompt("Number 2. What is 4 + 4?")
var questionThree = prompt("Ok, number 3. What is 5 x 5?")
var questionFour = prompt("Now, almost done. What is 4 - 3?")
var questionFive = prompt("last question! What is the capital of Idaho?")
// create conditionals to check the inputted answer from the user against the correct answer
// use parseInt() to check number value since when a user enters a value from prompt it will be a string
// question 5 is using toLowerCase() that way it will automatically convert their answer to all lowercase
// since we don't know if they will mix and match lower and uppercases
// also we use '===' because we want to compare their answer to the correct answer
if (parseInt(questionOne) === 4){
correctAnswers +=1
}
if (parseInt(questionTwo) === 8){
correctAnswers +=1
}
if (parseInt(questionThree) === 25){
correctAnswers +=1
}
if (parseInt(questionFour) === 1){
correctAnswers +=1
}
if (questionFive.toLowerCase() === 'boise'){
correctAnswers +=1
}
// Use an if/else so we can check what their prize is
// You can set this is multiple different way I'll explain why I did it this way
// First if checks for all 5 right. If true then alerts the winner of gold crown
// Else if is set for correctAnswers>= 3 . So if the correctAnswers is 3 or 4 then they will get a silver crown.
// We don't have to worry about it being 5 right because if it was 5 then it would have stopped at the first if with the gold crown
// Same deal with the second else if is set for correctAnswers >= 1 because it will cover if 1 or 2 are right
// Last but not least the `else` will cover if you have 0 right.
if (correctAnswers === 5){
alert('You received a gold crown')
} else if (correctAnswers >= 3){
alert('You received a silver crown')
} else if (correctAnswers >= 1){
alert('You received a bronze crown')
} else {
alert("I'm sorry but you didn't receive a crown")
}
Jeremy Leeper
2,013 PointsJeremy Leeper
2,013 PointsThank you so much for the feedback! Man do I have a long way to go. :-)