Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial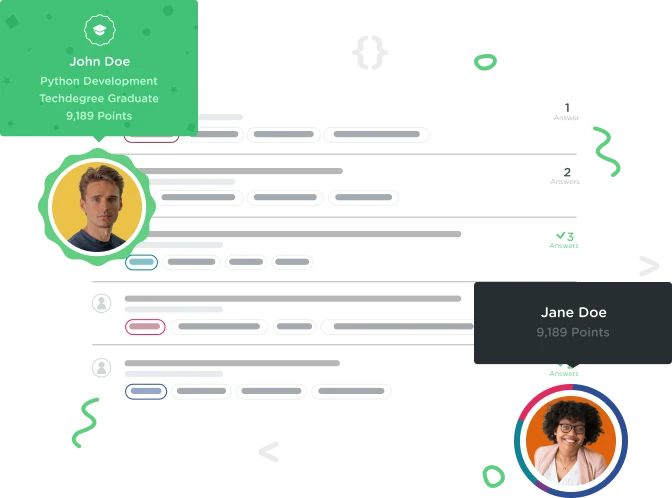

Marcela Jackowski
2,045 PointsHere's the full set of parameters coming in from a POST request again:
I cannot find help for this question. Thanks
class PetsController < ApplicationController
def show
@pet = Pet.find(params[:id])
end
def new
@pet = Pet.new
end
def create
pet_params = permit(:name)
@pet = Pet.new(pet_params)
@pet.save
end
end
Juan Luna Ramirez
9,038 PointsJuan Luna Ramirez
9,038 PointsRails saves any POST data to a params hash, in this case
http://guides.rubyonrails.org/action_controller_overview.html#parameters
Then, in order to get the pet hash
{ "name" => "Jessie" }
from params we need to require it like sopet_hash = params.require(:pet)
Now that we have the pet hash we can say which attributes are ok to update like so
permitted_pet_attributes = pet_hash.permit(:name)
Permitting is important to prevents accidentally allowing users to update sensitive model attributes. http://guides.rubyonrails.org/action_controller_overview.html#strong-parameters
Next we can instantiate a new pet object
@pet = Pet.new(permitted_pet_attributes)
Finally we save to the database
@pet.save
Of course you can chain methods together. I'll leave that up to you.