Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial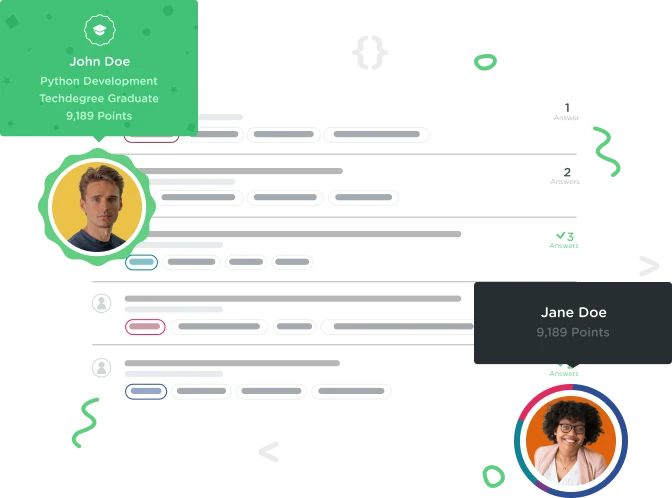

Marcin Lipski
9,947 PointsHey Can anyone find a problem with my code? For some reason preview mode doesnt work. Thanks
the preview doesnt work, so its is hard for me to see what is going on here. The hint message says, that html is wrong. Any suggestions?
<?php
$movie = array(
"title" => "The Empire Strikes Back",
"year"=> "1980",
"director"=>"Irvin Kershner",
"imdb_rating"=>"8.8",
"imdb_ranking"=>"11",
);
?>
<html>
<body>
<h1><?php echo $movie ["title"]; ?> (<?php echo $movie ["year"];?>)</h1>
<table>
<tr>
<th>Director</th>
<td><?php echo $movie ["director"];?></td>
</tr>
<tr>
<th>IMDB Rating</th>
<td><?php echo $movie ["imdb_rating"];?></td>
</tr>
<tr>
<th>IMDB Ranking</th>
<td><?php echo $movie ["imdb_ranking"];?></td>
</tr>
</table>
</body>
</html>
2 Answers
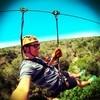
thomascawthorn
22,986 PointsHere's the working code:
<?php
$movie = [
'title' => 'The Empire Strikes Back',
'year' => '1980',
'director' => 'Irvin Kershner',
'imdb_rating' => '8.8',
'imdb_ranking' => 11,
];
?>
<h1><?php echo $movie['title'] ?> (<?php echo $movie['year'] ?>)</h1>
<table>
<tr>
<th>Director</th>
<td><?php echo $movie['director'] ?></td>
</tr>
<tr>
<th>IMDB Rating</th>
<td><?php echo $movie['imdb_rating'] ?></td>
</tr>
<tr>
<th>IMDB Ranking</th>
<td><?php echo $movie['imdb_ranking'] ?></td>
</tr>
</table>
A couple of VERY important things:
- There should be no space between your variable and the opening square bracket. Use $variable['key'] instead of $variable ['key'].
- Don't add additional mark up (unless asked!) to the code challenge - it sometimes looks for very specific output!
See how that goes :)

Marcin Lipski
9,947 PointsThanks guys. Haven't tried it yet, but your answer clarifies a lot.
hardieboeve
27,069 Pointshardieboeve
27,069 PointsYou miss the doctype and head declaration in your html, for example: