Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial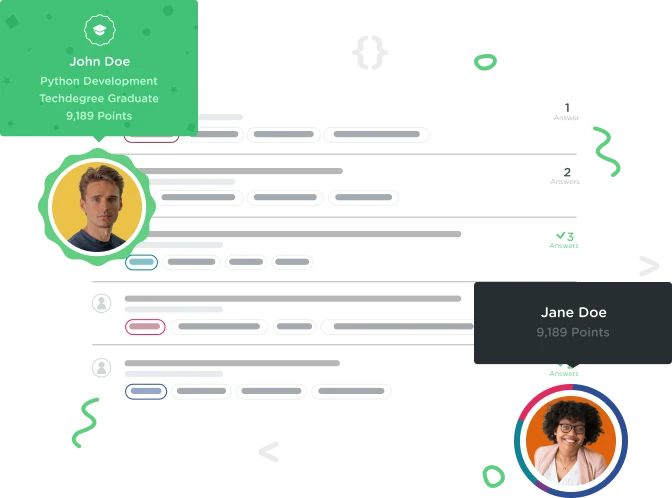

Rich TheDon
1,996 PointsHey! Dave said we should use parseInt() because prompt() is always a string. I have used Math.floor and it's working.
Is there a problem with using Math.floor()? What do you think?

Rich TheDon
1,996 Pointsvar randomNumber = (Math.floor(Math.random() * 6) + 1);
var guess = prompt("I am thinking of a number between 1 and 6. What is it?");
if (Math.floor(guess) === randomNumber) {
alert("You are right!");
} else {
alert("False!");
}

Antti Lylander
9,686 PointsIt seems that Math.floor() converts it's input to a number by itself. I just wonder why anyone would input a decimal in the prompt, i.e. why do you use Math.floor with guess in your conditional.
var randomNumber = (Math.floor(Math.random() * 6) + 1);
console.log(randomNumber + " " + typeof randomNumber)
var guess = prompt("I am thinking of a number between 1 and 6. What is it?");
console.log(guess + " " + typeof guess)
if (guess === randomNumber) {
alert("You are right!");
} else { alert("False!"); }
Try the above code ans open console and you'll see that variable quess is a string and randomNumber is a number.
3 Answers
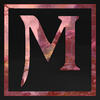
Matthew Lang
13,483 PointsJavaScript is a language which is "loosely typed", meaning it doesn't bother with data types too much. It does conversions automatically, for example:
let sum = "5" + 5;
// sum = 10
JavaScript converts the string to an integer in order to complete the calculation. This is why your Math.floor() function works on a string, too.
TypeScript on the other hand is a strict syntactical superset of JavaScript, which adds static typing. TypeScript transpiles into plain JavaScript. Read more about TypeScript here: https://www.typescriptlang.org/

tomd
16,701 PointsMath.floor is used for rounding down. While it does work, It is bad practice to use it for converting strings to numbers.
parseInt can also do things like this.
console.log(parseInt('100px')); // 100

Rich TheDon
1,996 PointsThanks for your replies. I don't think that it's odd to input a decimal if you get asked to write a number. This was the reason for me to use Math.floor().
Antti Lylander
9,686 PointsAntti Lylander
9,686 PointsPlease show your code.