Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial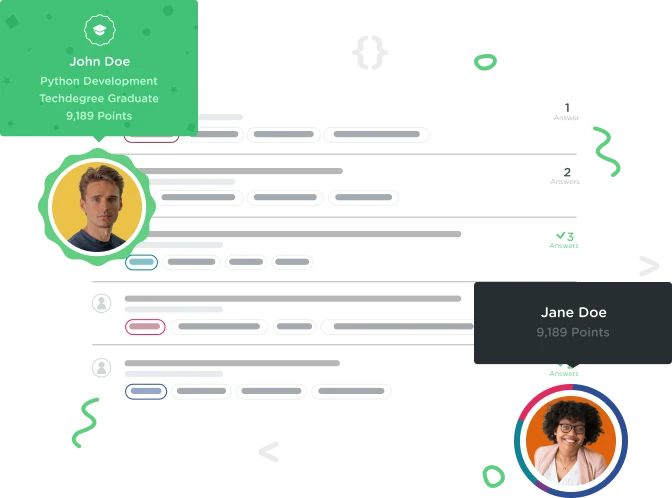

grctniu75rx
6,328 PointsHey guys, this isn't for a treehouse track, but I am curious about what am I doing wrong with this simple code in JS.
I have just dabbled in js and am struggling a bit
I want to write a function that accepts a name as input, and returns "Hello +name" also, if no valid name is inputted, the console should return "Hello?"
function sayHello() { var inputName; inputName = prompt("What is your name?"); console.log("Hello "+ inputName +"!"); while(inputName === null){ console.log("Hello?"); } }
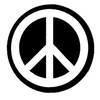
john larson
16,594 PointsThat's so weird, I just made some minor changes and it seems to work fine for me.
2 Answers

andren
28,558 PointsThe problem is that you use a "while" loop, you are telling java that you want it to keep console logging "hello?" while inputName is equal to null, and since you don't change inputName within the loop you end up with an infinite loop that never actually ends.
Also since the while statement comes after the first console.log that prints out "hello + inputName" that will always be ran even when inputName is null.
If you simply want to execute some code in one instance and some other code in another instance based on a condition then you should use an if/else statement instead. Like this:
if(inputName === null) {
console.log("Hello?");
} else {
console.log("Hello "+ inputName +"!");
}
With the above code you will get "hello?" printed if inputName is null and "Hello "+ inputName +"!" printed if inputName is anything else.
Another thing I would ask is how do you define "no name" currently you are only checking if the inputName variable is null, which it will only be if the name prompt is cancelled. I would personally also consider an empty answer to be invalid, but that won't be caught by simply checking for null.
Personally I would construct the if statement like this:
// This check if the inputName is null OR is empty
if(inputName === null || inputName.trim() === "") {
console.log("Hello?");
} else {
console.log("Hello "+ inputName +"!");
}
The trim function removes whitespace from the start and end of the string, so spaces at the beginning and end won't be counted for example, then it is compared to an empty string. This means that if you press enter without typing in anything, or just press the spacebar or something then your response will be counted as invalid.
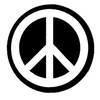
john larson
16,594 PointsWow Andren, did I miss that much? I was surprised to see the while loop where it was, but even more surprised that it seemed to do what stormy seemed to be intending it to.

andren
28,558 PointsAre you sure you actually ran the while loop? The while statement found in the original code causes an infinite loop if it actually runs, but it will only run if you cancel the question prompt that opens up. If you enter a name, or just leave the input blank but hit the "OK" button then the while loop will never start.
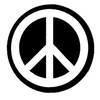
john larson
16,594 PointsJust some minor fixes. It works nicely :D
function sayHello() {
var inputName;
inputName = prompt("What is your name?");
console.log("Hello "+ inputName +"!");
while (inputName === null)
console.log("Hello?");
}
sayHello()
john larson
16,594 Pointsjohn larson
16,594 Points