Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial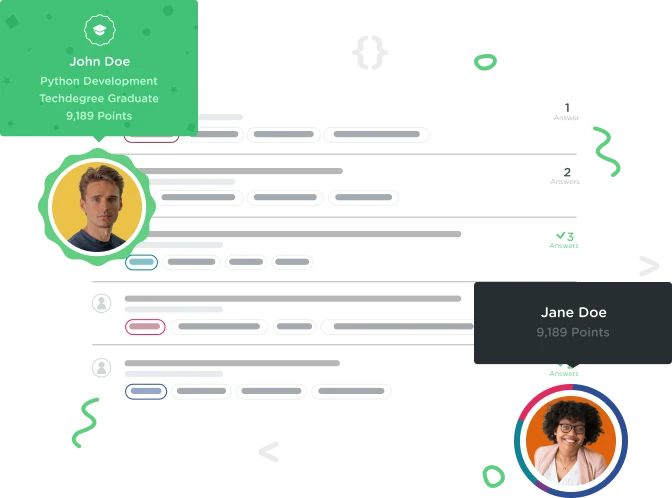

Rico Hodges-Smikle
Courses Plus Student 2,609 PointsHey, I'm a bit stuck on this section. Can I get some help with this code?
I can't seem to get this to pass, unclear what it means 'Can't get the length of 'Hand'
import random
class Die:
def __init__(self, sides=2):
if sides < 2:
raise ValueError("Can't have fewer than two sides")
self.sides = sides
self.value = random.randint(1, sides)
def __int__(self):
return self.value
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return self + other
class D20(Die):
def __init__(self, sides=0):
super().__init__(sides=20)
from dice import D20
class Hand(list):
@property
def total(self):
return sum(self)
@classmethod
def roll(cls,no_of_dice, *args, **kwargs):
die_rolled = Hand()
for _ in range(no_of_dice):
cls.append(die_rolled(D20))
return die_rolled
2 Answers
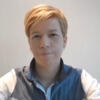
Ave Nurme
20,907 PointsHi Rico
The file dice.py seems to be OK, however there are issues in hands.py.
Here's my solution which I used & it passed, I've commented it for you:
from dice import D20 # import the D20 class
class Hand(list):
@property
def total(self):
return sum(self)
@classmethod # create a classmethod named roll
def roll(cls, dice_num): # take the number of dice as an argument
nums = [] # create an empty list
# in the following for-loop we append a D20 to our list equal to the
# number of dice given as an argument to the roll classmethod*
for _ in range(dice_num):
nums.append(D20())
return cls(nums) # return the list of D20s, classmethods need 'cls' here!
*For example, if we called Hand.roll(2)
then the number '2' in the parentheses would be the 'dice_num' in the def roll(cls, dice_num)
.
I hope this makes things a bit clearer for you!
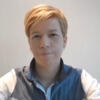
Ave Nurme
20,907 PointsThank you, Hermes!
Rico Hodges-Smikle
Courses Plus Student 2,609 PointsRico Hodges-Smikle
Courses Plus Student 2,609 PointsAwesome Thanks! I can see where I went wrong here.
Hermes d'Henares
2,756 PointsHermes d'Henares
2,756 PointsHi. Note that you should instantiate Hand instead of creating an empty list. That is, a more correct answer should be nums = Hand(). As Hand is a list, you are able to append dice to it.