Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial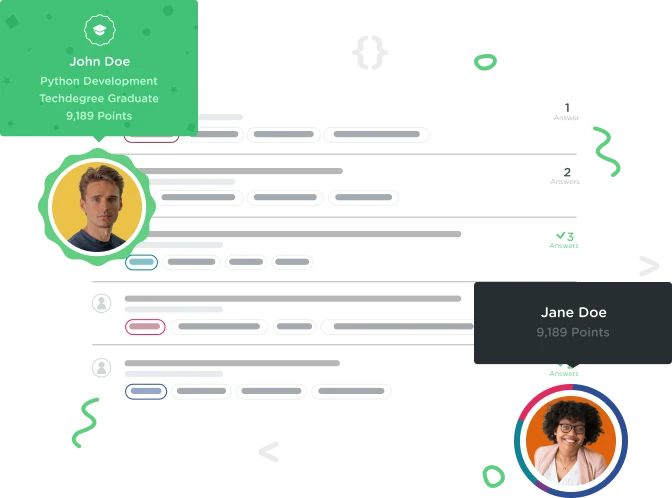

Tim Seme
1,664 Pointshey im super confused can u help me out
this is what it tells me to do but i dont fully understand
We also have three empty arrays, europeanCapitals, asianCapitals, and otherCapitals. The goal is to iterate through the dictionary and end up with just the names of the capital cities in the relevant array.
For example, after you execute the code you write, europeanCapitals will have the values ["Vaduz", "Brussels", "Sofia"] (not necessarily in that order).
To do this you're going to use a switch statement and switch on the key. For cases where the key is a European country, append the value (not the key!) to the europeanCapitals array. For keys that are Asian countries, append the value to asianCapitals and finally for the default case, append the values to otherCapitals.
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",// eur
"LIE": "Vaduz",// eur
"BGR": "Sofia",// eur
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",// eur
"IND": "New Delhi",// eur
"VNM": "Hanoi"]//asian
for (key, value) in world {
// Enter your code below
switch europeanCapitals.append(world){
case "BEL": print("Brusels")
case "LIE": print("Vaduz")
case "BGR": print("Sofia")
case "BRA": print("Brasilia")
case "IND": print("New Delhi")
}
switch asianCapitals.append(world){
case "VNM": print("Hanoi")
}
switch otherCapitals.append(world){
case "USA": print("Washington D.C.")
case "MEX": print("Mexico City")
}
// End code
}
1 Answer
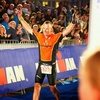
Steve Hunter
57,712 PointsHi Tim,
You want to switch
on the key
. Then, for each potential key, append value
into the relevant array. You do that inside each case
. You can handle multiple cases in one line.
Here's a little hint rather than the whole code:
for (key, value) in world {
// Enter your code below
switch(key){
case "BEL", "LIE", "BGR":
europeanCapitals.append(value)
break
.
.
}
// End code
}
I hope that helps,
Steve.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsTo clarify, the
for
loop is going through each member of theworld
dictionary. It parses over each element and makeskay
andvalue
available within the loop as local variables of the same name. At each iteration, you search for thkey
and, if found, youappend
it to the correct array. As you have used thekey
to identify the record you want to handle, youappend
the correspondingvalue
that thekey
gives you access to.Make sense?
Steve.