Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial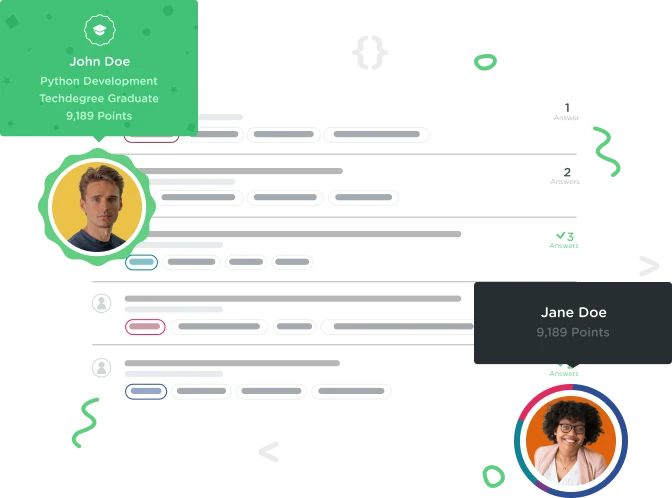
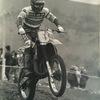
graemehunt
6,577 PointsHi all, please help, I'm really confused by a reference error: response is not defined -
I've changed my var to https, but it looks like something has changed in the docs from when the video was made - I've tried using all of the old 'response.on' code as well as the new and even mixed and matched a bit - but i seem to be stuck - can anyone help please?
// Look at a user's badge count and JS points
// Use node.js o connect Treehouse's API and print profile information.
var https = require("https");
var username = "graemehunt";
function printMessage(username, badgeCount, points) {
var message = username + " has " + badgeCount + " total badge(s) and " + points + " points in Javascript";
console.log(message);
}
// Connect to the API URL (https://teamtreehouse.com/username.json)
var request = https.get("https://teamtreehouse.com/" + username + ".json", function(response) {
console.log(response.statusCode);
});
// Read the data
response.on('data', function(chunk) {
console.log('BODY: ${chunk}');
});
// Parse the data
// Print the data
request.on("error", function(error){
console.error(error.message);
});
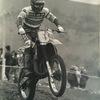
graemehunt
6,577 PointsHi thank you christianandersson, my first post with code - I should have checked the cheatsheet first!

Christian Andersson
8,712 PointsAh, that's better! :)
1 Answer

Christian Andersson
8,712 PointsYou're getting an error telling you that the variable response
is undefined - basically that it's an unknown variable that hasn't been declared before.
This is because response
was used as the argument in your callback function and is scoped to that function (i.e it's only "visible" inside that function, but not outside).
I believe your code should work if you move some of your code inside your callback, rather than outside:
// Look at a user's badge count and JS points
// Use node.js o connect Treehouse's API and print profile information.
var https = require("https");
var username = "graemehunt";
function printMessage(username, badgeCount, points) {
var message = username + " has " + badgeCount + " total badge(s) and " + points + " points in Javascript";
console.log(message);
}
// Connect to the API URL (https://teamtreehouse.com/username.json)
var request = https.get("https://teamtreehouse.com/" + username + ".json", function(response) {
console.log(response.statusCode);
// Read the data
response.on('data', function(chunk) {
console.log('BODY: ${chunk}');
});
// Parse the data
// Print the data
}); //<---------- callback ends here
request.on("error", function(error){
console.error(error.message);
});
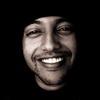
miikis
44,957 PointsAlmost. The request.on()
part should be outside of the https.get()
, after the request
. This way, you're not calling request#on() every time you get a response.

Christian Andersson
8,712 PointsOh yeah, I thought it was another response.on
thingy - words too similar :D. Thanks.
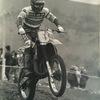
graemehunt
6,577 Pointsexcellent! thank you very much for your help - that seems to have done the trick : - )
Christian Andersson
8,712 PointsChristian Andersson
8,712 PointsHey graemehunt, could you please edit your post and format the code so that it's more readable? Right now it's just a blob and hard to read :)