Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial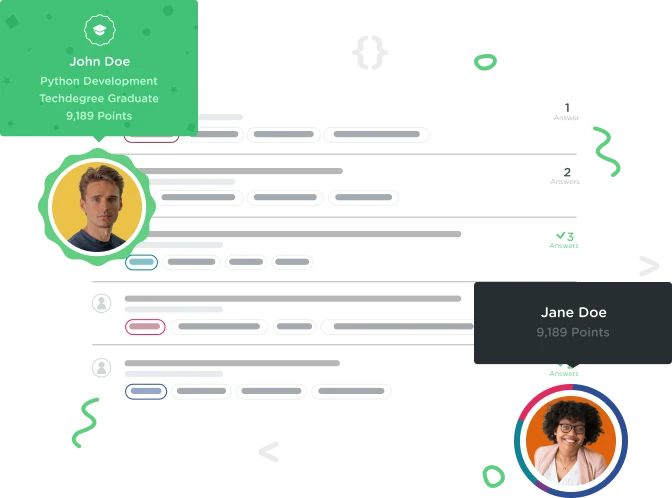

Dairon Castellanos
1,043 PointsHi am in java basic and I really want to know how can I export and use the java.io.console on eclipse.
I really need to know how to export the java io console in eclipse or any other text editor outside of TreeHouse so I can practise what I learng.
3 Answers
Christopher Augg
21,223 PointsHeya All,
It is my understanding that java.io.Console has had a reported issue with Eclipse and other IDE's for about 9 years. According to the Eclipse bugs site at https://bugs.eclipse.org/bugs/show_bug.cgi?id=122429 , there has been no official fix. However, there are a few work arounds.
The reason for the issue is due to how the class Console works. The documentation at: http://docs.oracle.com/javase/7/docs/api/java/io/Console.html states, "Whether a virtual machine has a console is dependent upon the underlying platform and also upon the manner in which the virtual machine is invoked. If the virtual machine is started from an interactive command line without redirecting the standard input and output streams then its console will exist and will typically be connected to the keyboard and display from which the virtual machine was launched. If the virtual machine is started automatically, for example by a background job scheduler, then it will typically not have a console.
If this virtual machine has a console then it is represented by a unique instance of this class which can be obtained by invoking the System.console() method. If no console device is available then an invocation of that method will return null. "
Therefore, when developing in Eclipse or other IDE, you import java.io.Console and invoke System.console(), it will return null because the virtual machine is not started from an interactive command line. On the contrary, if you use Terminal (OSX) or command prompt(Windows), your code will work fine by using the javac and java commands to compile and run your code. Of course, if you have to use a terminal/command prompt, then you would not want to use Eclipse. You would be better off using a text editor like notepad, Notepad++, Sublime Text 2, Nano, VIM, Emacs, etc.
One work around is given at the end of the bug comments via the first link I provided above. However, it is a hack that anyone here new to java and IDE's might not be comfortable completing. Personally, I prefer to avoid console altogether anyhow. Which leads to another work around.
Another work around for output is to use System.out.printf() when you need to pass arguments as you did with console.printf(). Although, whenever you do not need to pass arguments, then use System.out.println() or System.out.print() for the purposes of efficiency. As for input, you can use the Scanner class. You can use these very easily when following the Java track here by just replacing the console code.
Example:
import java.util.Scanner;
public class MAIN {
public static void main(String[] args) {
// Print something when not passing arguments.
System.out.println("Please enter your first name: ");
// Instantiate Scanner object and wait for user input.
Scanner input = new Scanner(System.in);
// Assign the String given by the user to firstName.
String firstName = input.nextLine();
// Print something when passing arguments.
System.out.printf("Hello %s ", firstName);
// Close the Scanner when done with it.
input.close();
}
}
There are other work arounds as well. Just google around and you will find them.
With all that said, I highly recommend that anyone getting started with programming refrain from using an IDE if you plan on applying for jobs with the skills you are learning. Interviews require you to write code on whiteboards or in plain text editors while screen sharing. If you learn to code with an IDE, you will most likely not remember a lot of the syntax due to always allowing auto complete to finish things. Nor will you train yourself to find even the simplest of errors in your code. You can turn a lot of these features off, but why use an IDE without features? Using the Treehouse workspace, your systems terminal, or your systems command prompt are all good ways to get started. You can always move to an IDE once you are done learning the basics here. Craig Dennis even provides a course here for doing that with IntelliJ.
Regards,
Chris
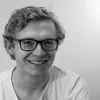
Luke Glazebrook
13,564 PointsHi Dairon!
To use 'java.io.console' you will need to use an import statement to include it in your project. You can do this by including the following line at the top of your project.
import java.io.Console;
I hope this helped you out!
-Luke
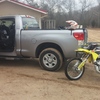
Ryan Ruscett
23,309 PointsHey,
You don't have to export the Java.io.console in order to use it in eclipse.
You need to go into eclipse and just type import java.IO.* or import java.io.console
this packages is included in java. No need to do anything else to use it. Just import it into your class.
Ryan Ruscett
23,309 PointsRyan Ruscett
23,309 PointsHey, thanks for the information. I do use console in eclipse Kepler and eclipse mars on Ubuntu, as well as in java repl. I did take a look at those bugs and I didn't realize so many other individuals have had issues with it. So there may be a trick to it for some.
Thanks!
Christopher Augg
21,223 PointsChristopher Augg
21,223 PointsInteresting Ryan,
I have never tried it under Ubuntu before. Therefore, I figured I would attempt it to see how it goes. Unfortunately, I still get null returned from System.console(); I did this with Eclipse Mars on Ubuntu 14.04 using Java 8 sdk on virtual box.
Do you recall the install procedure you used? I used apt-get for all installations within the terminal. We might be able to figure out a solution for other operating systems if we find out the procedure you followed that allows Eclipse to have a console returned from System.console();
Regards,
Chris
Dairon Castellanos
1,043 PointsDairon Castellanos
1,043 PointsThanks a lot