Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial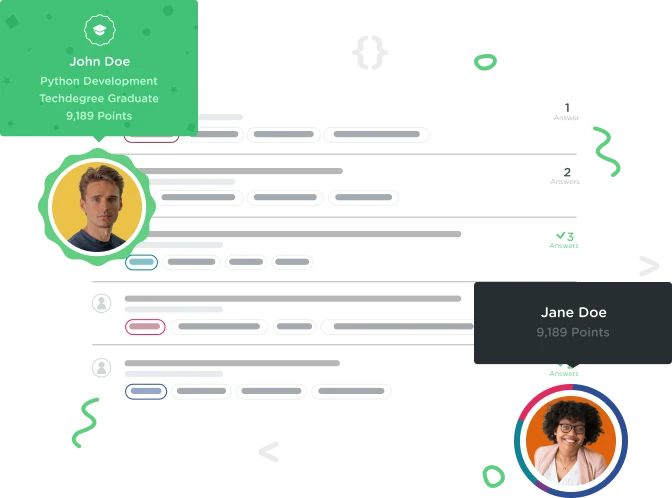
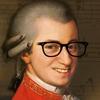
Adam Balogh
1,307 Pointshi can somebody check out this code? https://w.trhou.se/ah34ypyhng
I just want to know if there are more better methods to write this code
3 Answers

Cooper Runstein
11,850 PointsYour code is good, and until you take a more advanced course, this is probably the best way to accomplish this. I'd recommend you take the JS DOM manipulation course where you'll learn how to use html to take inputs rather than the alert box.
As far as the code you have now, I have a few recommendations that are more for the sake of readability than functionality:
var message =
"<h1>Math with the numbers " +firstNumber+ " and " +secondNumber+"</h1>" + //h1 should only be used for titles, not for the entire block of html
"<div>" + firstNumber + "+" + secondNumber + " = " + plus + "</div>" + //Div, p, or span tags are more appropriate here
"<div>" +firstNumber+ "-" +secondNumber+ "=" +minus+"</div>" +
"<div>" +firstNumber+ "X" +secondNumber+ "=" +multi+"</div>" +
"<div>" +firstNumber+ "/" +secondNumber+ "=" + div + "</div>";
document.write(message); //Your previous variable declaration wasn't doing anything, either use document.write on the string, or declare and create the variable, and then use it as I did here.
Overall, nice job, learning about DOM manipulation and ES6 template literals will improve this a lot, so I'd recommend you get work your way up to that, the full stack JS track is a good place to learn all of that. Good luck!
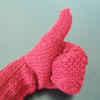
Jonathan Mitten
Courses Plus Student 11,197 PointsThere are definitely ways to write this in a more organized fashion, but functionally speaking, they're going to provide the same output. Since your example is really simple, this is not so much a suggestion as just something to look forward to:
var multi = function(){
let product = firstNumber * secondNumber;
return `${firstNumber} * ${secondNumber} = ${product} <br>`;
}
var add = function(){
let sum = firstNumber + secondNumber;
return `${firstNumber} + ${secondNumber} = ${sum} <br>`;
}
var subtract = function(){
let difference = firstNumber - secondNumber;
return `${firstNumber} - ${secondNumber} = ${difference} <br>`;
}
var divide = function(){
let quotient = firstNumber / secondNumber;
return `${firstNumber} / ${secondNumber} = ${quotient} <br>`;
}
var div = parseFloat(firstNumber)/parseFloat(secondNumber);
var minus = parseFloat(firstNumber)-parseFloat(secondNumber);
var plus = parseFloat(firstNumber)+parseFloat(secondNumber);
var message = ("<h1>Math with the numbers " +firstNumber+ " and " +secondNumber+"<br>"
+ add()
+ subtract()
+ multi()
+ divide() + "</h1>");
document.write(message);
By separating out the functionality from the document.write()
output, you can reuse the functionality elsewhere. Now, in this case, I include the "<br>" code in the return statement, which isn't really that useful for reusability, but you can see that it's possible to blend string text and raw variables together by using the javascript Template Literal technique, making it much easier to read when you come back to it. Even though I wrote this, I did so as just one example of what you could do. If I were to review my own work, I would remove the <br>
from the functions and put them back where you had them, or write another function that runs to tack on a <br>
to the end of one of the 4 main functions.
You can also use template literals in the document.write() message, which would remove the need to concatenate with plus-signs, like this:
var message = `<h1>Math with the numbers ${firstNumber} and ${secondNumber}<br>
${add()}
${subtract()}
${multi()}
${divide()} </h1>`;
document.write(message);
Let me know if this makes any sense, or if it's even what you're looking for in an answer.
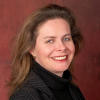
Niki Molnar
25,698 PointsFor the exercise, I would parseFloat() each number as you get it, so you donβt have to keep repeating parseFloat() all the time. For example:
var firstNumber = prompt("Give me any number please ");
firstNumber = parseFloat(firstNumber);
var secondNumber = prompt("another one please.");
secondNumber = parseFloat(secondNumber);
Then you can use:
firstNumber + secondNumber
without having to pass them through parseFloat() each time.