Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial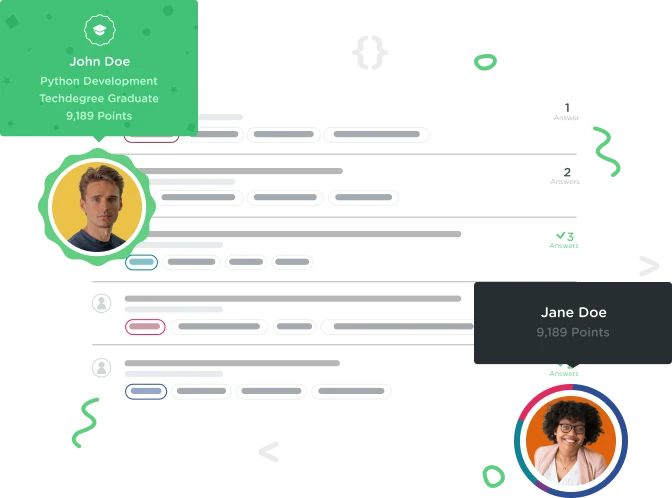

Modestas Kiudulas
1,590 PointsHi, can somebody check what is wrong with my Program and provide feedback? Thanks!
// Question 1 var score = 0; var q1 = prompt("How many days in a year?");
if (q1 == 365) { alert("Correct!"); document.write("<p>Question 1 Correct</p>"); score +=1; }
else { alert("Incorrect!"); document.write("<p>Question 1: Incorrect</p>"); }
// Question 2 var q2 = prompt("What is the closest planet to the Sun?");
if (q2.toUpperCase === "MERCURY") { alert("Correct!"); document.write("<p>Question 2: Correct</p>"); score +=1 ; }
else { alert("Incorrect!"); document.write("<p>Question 2: Incorrect</p>"); }
// Question 3
var q3 = prompt("What is 2 x 2?");
if (q3.toUpperCase == 4 || q3 === "FOUR" ) { alert("Correct!"); document.write("<p>Question 3: Correct</p>"); score +=1; }
else { alert("Incorrect!"); document.write("<p>Question 3: Incorrect</p>"); }
// Question 4 var q4 = prompt("what is the biggest animal on the planet?");
if (q4.toUpperCase === "BLUE WHALE" || q4.toUpperCase === "BLUEWHALE" || q4.toUpperCase === "WHALE") { alert("Correct!"); document.write("<p>Question 4: Correct</p>"); score +=1; } else { alert("Incorrect!"); document.write("<p>Question 4: Incorrect</p>"); }
// Question 5 var correctAnswer = false var q5 = prompt("Is Pluto a Planet?");
if (q5.toUpperCase === "NO") { correctAnswer = true; }
if (correctAnswer) { alert("Correct!"); document.write("<p>Question 5: Correct</p>"); score +=1; }
else { alert("Incorrect!"); document.write("<p>Question 5: Incorrect</p>"); }
//Final Score and Message if (score === 5) { document.write("<p>Your Total Score is </p>" + score + "<p>!</p>" + "<p>Congratulations, you get a Gold Medal!</p>"); }
else if (score === 4 || score === 3) { document.write("<p>Your Total Score is </p>" + score + "<p>!</p>" + "<p>Congratulations, you get a Silver Medal!</p>"); }
else if (score === <3>=1) { document.write("<p>Your Total Score is </p>" + score + "<p>!</p>" + "<p>Congratulations, you get a Bronze Medal!</p>"); }
else { document.write("<p>Unfortunately, your Total Score is </p>" + score + "<p>Better Luck next time!</p>"); }
6 Answers

Modestas Kiudulas
1,590 PointsThe code isn't running when i preview it. It's just a blank page. And yes it is linked to the HTML page.

Anthony McCormick
6,774 PointsThe reason your code is not running running due to the way you have set up your conditional statement. See your code below:-
else if (score === <3>=1) { document.write("<p>Your Total Score is </p>" + score + "<p>!</p>" + "<p>Congratulations, you get a Bronze Medal!</p>"); }
This is incorrect. You do not need the equality operator. See mine below:-
else if (score < 3 && score >= 1) {
document.write("<p>Your Total Score is </p>" + score + "<p>!</p>" + "<p>Congratulations, you get a Bronze Medal!</p>");
}

Modestas Kiudulas
1,590 PointsThanks, my code is running now. Although there is still a slight issue. regardless of my answer, every question apart from the first one seems to give me the "incorrect" alert. do you know what may be causing this?

Anthony McCormick
6,774 PointsI'd recommend trying to figure it out yourself and if you're still struggling, to come back. :)

D P
3,503 PointsOne issue is that your line:
if (q3.toUpperCase == 4 || q3 === "FOUR" )
isn't quite what you need. It should be:
if(parseInt(q3) === 4 || q3.toUpperCase() === "FOUR")
Remember that prompt() returns a String, so to compare whatever it returns to a Number you need to use parseInt() to convert it to a Number first.

Modestas Kiudulas
1,590 PointsHmm.. but i thought that the double equality operator can be sued to match a string and a number or am i mistaken?

D P
3,503 PointsThe double equals operator can be used to confirm that "4" == 4 but not "FOUR" == 4.
Also, there's no reason to use toUpperCase() with a Number as Numbers don't have cases.
Anthony McCormick
6,774 PointsAnthony McCormick
6,774 PointsWhat is the exact problem you are having?