Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial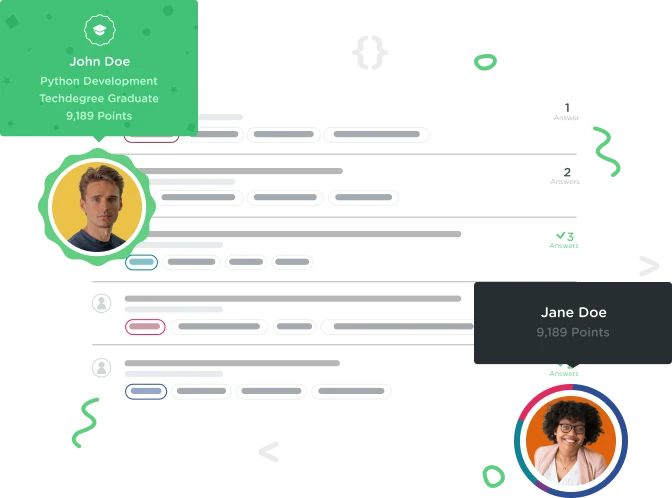

nagamani palamuthi
6,160 PointsHi , can someone help me understand this concept of lastevent and setting it equal to e?
what does the e repesent and how does setting the offsets X and Y create the mouse move. that whole part of lastevent and the e is confusing to me.
3 Answers
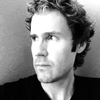
Lee Zamastil
21,628 PointsThe answer lays, in part, in the jQuery documentation for the .mousedown() method. Though I don't see an example that uses the e
parameter, it does mention that "An object containing dataβ¦will be passed to the event handler."
$canvas.mousedown(function(e) {
lastEvent = e;
...
e
is the event object. By convention it is often named e
. It could reasonably be named event
. But, being like a variable, it could even be named squirrel
. e
is just a common choice as shorthand for "event object".
Every event handling function receives an event object (whether you want it to or not β it's automatic). If you have no use for the event object then you could simply leave the parentheses following the function
keyword empty:
$canvas.mousedown(function() {
...
At 3:32 in the video you can see Andrew C. display the contents of this MouseEvent object in the JavaScript Console of the Developer Tools. You may note that two of the object's properties are offsetX
and offsetY
.
There are essentially two event objects at work in the following code:
//On mouse events on the canvas
$canvas.mousedown(function(e) {
lastEvent = e;
mouseDown = true;
}).mousemove(function(e){
//Draw lines
if(mouseDown) {
context.beginPath();
context.moveTo(lastEvent.offsetX, lastEvent.offsetY);
context.lineTo(e.offsetX, e.offsetY);
context.strokeStyle = color;
context.stroke();
lastEvent = e;
}
...
They are both assigned to e
. This works because in the first event,.mousedown()
, the contents of e
(again, the event object containing offsetX
and offsetY
properties ) are immediately assigned to the global variable lastEvent
. Then, when you move the mouse and trigger the second event, .mousemove()
, a new event object is created and assigned to e
in this line:
}).mousemove(function(e){
This second event object simply remains as e
.
So the line:
context.moveTo(lastEvent.offsetX, lastEvent.offsetY);
is the precise point where the .mousedown()
was triggered β where you clicked your mouse.
And the line:
context.lineTo(e.offsetX, e.offsetY);
is the precise point where the .mousemove()
was triggered β the spot to where you moved the mouse pointer.
Then, the canvas
element does its HTML5 magic and draws connecting lines between those offsets. And it keeps doing it as long as you keep moving the mouse and keep triggering .mousemove()
. This creates the shapes you draw on screen.
Clear, n'est-ce pas?

Iain Simmons
Treehouse Moderator 32,305 Pointse
in this case will be equal to the event parameter that is passed in, which would be a MouseEvent.
It has a number of properties that the browser will record, update and make available for scripts to use.

nagamani palamuthi
6,160 Pointsthank you so much for you detailed explanation. Lee... helped me comprehend better. thanks Iain . that was helpful too!
Nando Delgado
7,157 PointsNando Delgado
7,157 PointsI was having the same doubt, thanks for the great answer.
Ivo Vieira
5,443 PointsIvo Vieira
5,443 PointsThanks a lot! Your detailed answer was a great help. If you allow me, I'm still having just a wee doubt: so .moveTo(...) is the precise point where the .mousedown() was triggered and .lineTo(...) the spot where we moved the mouse pointer to. Wouldn't make more sense '.moveTo(...)' being called '.moveFrom(...) since - just be sure that I'm understanding it correctly - our starting point?